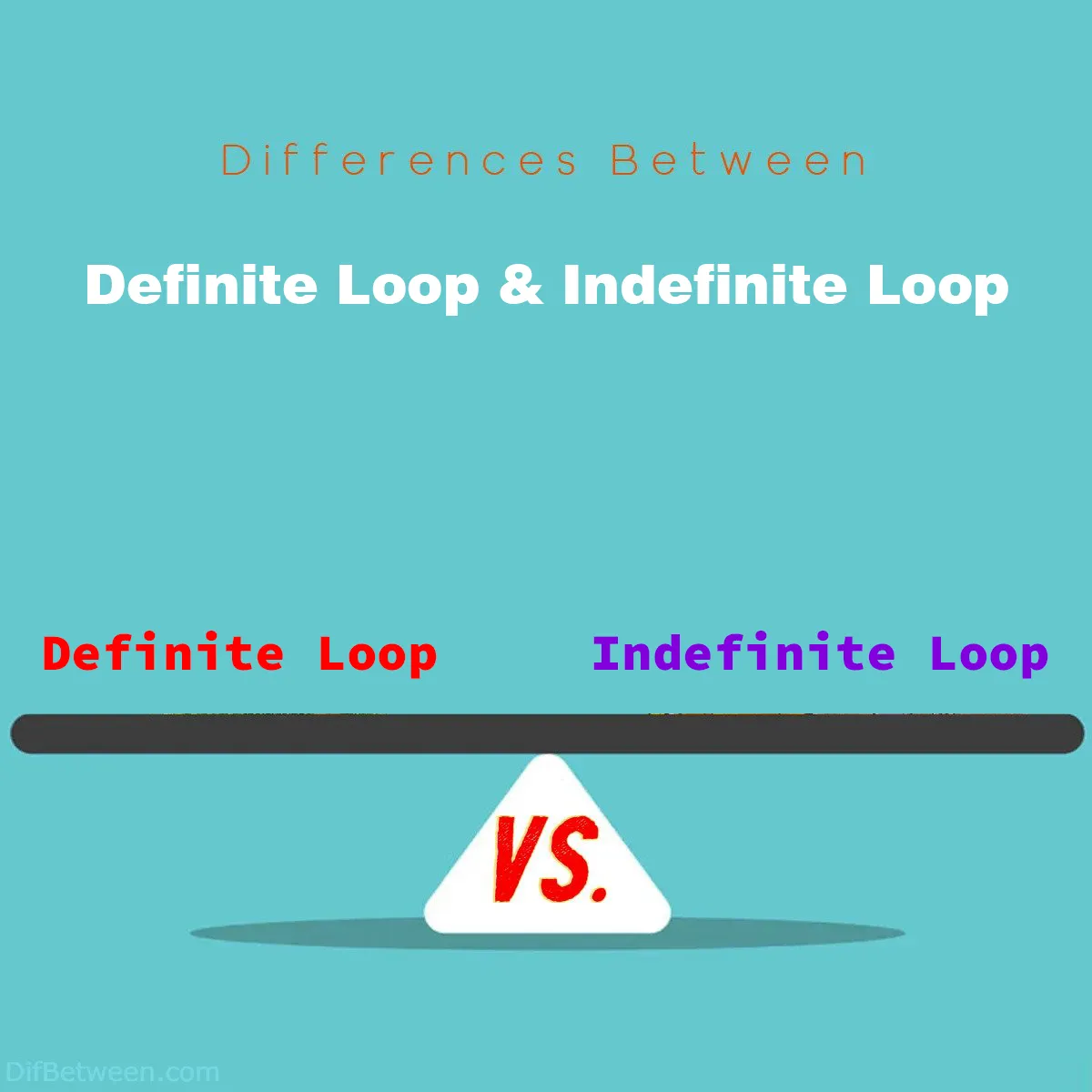
Aspect | Definite Loops | Indefinite Loops |
---|---|---|
Control Flow | Follows a predetermined number of iterations. | Continues executing as long as a condition holds. |
Iteration Count | Known and fixed in advance. | Unknown and depends on runtime conditions. |
Ideal Use Cases | – Iterating through fixed-size data structures. – Countdowns and timers with a known duration. – Batch processing of data. – Data validation with a fixed number of checks. | – Handling user input and menu-driven interfaces. – Managing server requests with variable response times. – Real-time systems reacting to dynamic events. |
Syntax Examples | – Python: for i in range(5) <br>- Java: for (int i = 0; i < 5; i++) <br>- C++: for (int i = 0; i < 5; i++) | – Python: while True: <br>- Java: while (true) <br>- C++: while (true) |
Performance Considerations | Typically more efficient due to a fixed number of iterations. Compiler optimizations are possible. | Less predictable due to runtime-dependent conditions. May be less efficient if not designed carefully. |
Common Pitfalls | – Off-by-one errors in iteration count. – Array indexing errors. – Inflexibility in handling dynamic scenarios. | – Infinite loops without proper exit conditions. – Incorrect or ambiguous exit conditions. – Risk of excessive resource consumption. |
In the world of programming, loops are like the unsung heroes that help us automate repetitive tasks and make our code more efficient. There are primarily two types of loops: definite and indefinite loops. Each type serves a unique purpose and has its own set of characteristics. In this comprehensive guide, we’ll delve into the key differences between definite and indefinite loops, shedding light on when and how to use them in your code.
Differences Between Definite Loop and Indefinite Loop
The main differences between definite loops and indefinite loops lie in their control flow and iteration count. Definite loops, as the name suggests, have a predetermined and known number of iterations, providing precise control over execution and making them suitable for tasks with fixed requirements. In contrast, indefinite loops operate as long as a condition remains true, accommodating dynamic scenarios and adapting to runtime conditions. The choice between the two depends on the specific needs of a programming task, with definite loops favored for predictability and performance, while indefinite loops excel in situations where iteration count is uncertain and adaptability is key.
1. Definite Loops vs. Indefinite Loops: An Introduction
Definite Loops
Definite loops, as the name suggests, have a clearly defined and known number of iterations. These loops are your go-to choice when you want to repeat a set of instructions a specific number of times. In programming, they are also known as “for” loops in languages like Python, Java, and C++.
Characteristics of Definite Loops:
- Known Iteration Count: You know exactly how many times the loop will execute.
- Controlled Termination: The loop terminates when the predefined condition is met.
- Ideal for Arrays: Definite loops are often used for iterating through arrays, lists, or collections.
Indefinite Loops
Indefinite loops, on the other hand, don’t have a predetermined number of iterations. They continue to execute as long as a certain condition remains true. While these loops can be incredibly flexible, they require careful handling to avoid infinite loops that can crash your program.
Characteristics of Indefinite Loops:
- Unknown Iteration Count: The number of iterations depends on runtime conditions.
- Dynamic Control Flow: The loop continues until the condition becomes false.
- Great for User Input: Indefinite loops are commonly used for handling user input where the number of iterations is uncertain.
Now that we have a basic understanding of the two loop types, let’s explore the key differences between them in more detail.
2. Control Flow: The Fundamental Difference
At the core, the primary distinction between definite and indefinite loops lies in how they handle control flow.
Definite Loop Control Flow
Definite loops have a straightforward control flow. They start with an initialization step and then repeatedly execute a set of instructions until a specified condition evaluates to false. Once the condition is no longer met, the loop terminates.
Here’s a simplified flowchart of a definite loop:
Start --> Initialization --> Condition Check --> [Condition True] --> Loop Body --> [Condition False] --> End
In this flowchart, the loop starts by initializing any necessary variables, then checks the condition. As long as the condition is true, it continues to execute the loop body, and when the condition becomes false, it exits the loop.
Indefinite Loop Control Flow
Indefinite loops, on the other hand, have a more dynamic control flow. They start with an initial condition check, and if the condition is true, they enter the loop body. The loop continues to execute the body until the condition evaluates to false, at which point it exits.
Here’s a simplified flowchart of an indefinite loop:
Start --> Initial Condition Check --> [Condition True] --> Loop Body --> [Condition Check Again] --> [Condition False] --> End
In this flowchart, the loop begins with an initial condition check. If the condition is true, it proceeds to execute the loop body. After each iteration, it checks the condition again. If the condition is still true, it continues, and if it becomes false, the loop ends.
3. Iteration Count: Known vs. Unknown
Another crucial difference between definite and indefinite loops is whether you know the number of iterations in advance or not.
Definite Loop Iteration Count
With definite loops, you have complete knowledge of the number of iterations from the outset. This can be advantageous when you need to repeat a specific task a predetermined number of times. It allows for precise control over the loop’s behavior.
Pros of Known Iteration Count (Definite Loops):
- Predictable behavior: You can anticipate how many times the loop will run.
- Ideal for fixed-size data structures: When dealing with arrays or lists of known sizes, definite loops shine.
Cons of Known Iteration Count (Definite Loops):
- Limited flexibility: Definite loops are not well-suited for situations where the number of iterations is uncertain.
Indefinite Loop Iteration Count
Indefinite loops, as the name suggests, have an unknown number of iterations. This makes them incredibly flexible but also requires careful handling to prevent unintended infinite loops.
Pros of Unknown Iteration Count (Indefinite Loops):
- Adaptability: Indefinite loops are perfect for scenarios where the number of iterations depends on user input or dynamic conditions.
- Robustness: They can handle varying data sizes without modification.
Cons of Unknown Iteration Count (Indefinite Loops):
- Complexity: Writing and maintaining code for indefinite loops can be more challenging due to the uncertain nature of their iterations.
- Risk of infinite loops: If not properly controlled, indefinite loops can lead to programs that hang or crash.
4. Use Cases: When to Choose Which
Now that we’ve covered the fundamental differences, let’s explore the scenarios where you should choose definite loops or indefinite loops based on their strengths.
Scenarios for Definite Loops
- Iterating Through Arrays: When you have a fixed-size array or list, and you need to perform an operation on each element, a definite loop is the way to go. You can precisely control the number of iterations, ensuring every element is processed.
- Countdowns and Countdown Timers: When you need to create countdowns or timers with a known duration, definite loops excel. For example, implementing a countdown from 10 to 1.
- Batch Processing: In scenarios where you need to process data in fixed-size chunks, definite loops offer a clear advantage. For instance, splitting a large dataset into manageable portions for analysis.
- Data Validation: When you want to validate user input a specific number of times, such as validating a password or PIN, definite loops provide a structured approach.
Scenarios for Indefinite Loops
- User Input Handling: Indefinite loops are a natural choice when dealing with user input. They allow your program to continue gathering data until a specific exit condition is met.
- Server Requests: In network programming, you often deal with unpredictable response times. Indefinite loops can help you retry requests until a successful response is received or a timeout occurs.
- Real-time Systems: When building systems that need to react in real-time to external events (e.g., sensor data), indefinite loops can continuously monitor and respond to changes.
- Menu Driven Programs: Applications with menu-driven interfaces often use indefinite loops to repeatedly display options until the user chooses to exit.
In summary, the choice between definite and indefinite loops depends on the nature of your task and the level of control you require over the loop’s execution.
5. Syntax Comparison: How They Look
Let’s now take a closer look at the syntax of definite and indefinite loops in some popular programming languages.
Syntax of Definite Loops
Python (for loop)
for i in range(5): print("This is iteration", i)
Java (for loop)
for (int i = 0; i < 5; i++) { System.out.println("This is iteration " + i); }
C++ (for loop)
for (int i = 0; i < 5; i++) { cout << "This is iteration " << i << endl; }
Syntax of Indefinite Loops
Python (while loop)
while True: user_input = input("Enter 'quit' to exit: ") if user_input == 'quit': break
Java (while loop)
import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); while (true) { System.out.print("Enter 'quit' to exit: "); String userInput = scanner.nextLine(); if (userInput.equals("quit")) { break; } } } }
C++ (while loop)
#include <iostream> #include <string> using namespace std; int main() { string userInput; while (true) { cout << "Enter 'quit' to exit: "; getline(cin, userInput); if (userInput == "quit") { break; } } return 0; }
These examples illustrate the basic structure of definite and indefinite loops in Python, Java, and C++. In definite loops, you specify the number of iterations in the loop header. In indefinite loops, you use a control condition to determine when the loop should exit.
6. Performance Considerations: Efficiency Matters
Efficiency is a critical factor in programming, and the choice between definite and indefinite loops can impact your program’s performance.
Performance of Definite Loops
Definite loops are generally more efficient when it comes to performance. Since you know the exact number of iterations in advance, the compiler or interpreter can optimize the loop’s execution.
Pros of Definite Loop Performance:
- Predictable runtime: The execution time of definite loops is known and constant.
- Compiler optimizations: Compilers can often optimize definite loops for better performance.
Cons of Definite Loop Performance:
- May not be suitable for dynamic situations: If the number of iterations changes based on runtime conditions, definite loops can be less efficient.
Performance of Indefinite Loops
Indefinite loops, by their nature, are less predictable in terms of performance. They continue to execute until a condition is met, which means the runtime can vary significantly.
Pros of Indefinite Loop Performance:
- Flexibility: Indefinite loops are well-suited for dynamic scenarios, allowing your program to adapt to changing conditions.
Cons of Indefinite Loop Performance:
- Potential for inefficiency: If not designed carefully, indefinite loops can lead to unnecessary computations and decreased performance.
- Risk of infinite loops: Inadequate control conditions can result in infinite loops, which can consume CPU resources and hang your program.
When choosing between definite and indefinite loops, consider the trade-off between control and efficiency. If performance is critical and the number of iterations is known, definite loops are often the better choice. However, if you need flexibility and adaptability, indefinite loops may be necessary despite potential performance variations.
7. Common Pitfalls: Watch Out for These
Regardless of whether you’re using definite or indefinite loops, there are common pitfalls to be aware of.
Pitfalls with Definite Loops
- Off-by-One Errors: In definite loops, it’s easy to make off-by-one errors when defining the iteration count. Ensure your loop condition is set correctly to avoid missing the last iteration or running one extra iteration.
- Array Indexing Errors: When iterating through arrays or lists, be cautious with indexing. Accessing elements outside the valid range can lead to runtime errors.
- Inflexibility: Definite loops are not suitable for scenarios where the number of iterations is uncertain. Using them in such situations can result in code that doesn’t work as expected.
Pitfalls with Indefinite Loops
- Infinite Loops: The most significant risk with indefinite loops is creating infinite loops that never exit. Always provide a clear exit condition to prevent your program from becoming unresponsive.
- Incorrect Exit Conditions: Ensure your exit conditions are well-defined and accurate. Incorrect conditions can cause your program to exit prematurely or not at all.
- Resource Consumption: Be mindful of resource consumption in indefinite loops, especially in long-running programs. Continuous execution can lead to high CPU usage and memory leaks if not managed properly.
Definite Loop or Indefinite Loop : Which One is Right TO Choose?
Choosing between a definite loop and an indefinite loop depends on the specific requirements of your programming task and the nature of the problem you are trying to solve. Both types of loops have their own strengths and weaknesses, and the decision should be based on the characteristics of your problem and your programming goals.
When to Choose a Definite Loop:
- Known Iteration Count: Use definite loops when you know in advance how many times you need to repeat a set of instructions. This is especially useful for tasks that require a fixed number of iterations.
- Precise Control: Definite loops provide precise control over the loop’s execution. You can anticipate and control the exact number of iterations, which can be crucial for tasks like array processing or countdowns.
- Performance-Critical Scenarios: In situations where performance is critical and you want to minimize runtime variability, definite loops are often the better choice. Compiler optimizations can be applied more effectively to these loops.
- Handling Fixed-Size Data Structures: When working with fixed-size data structures like arrays or lists, definite loops are well-suited for iterating through their elements.
When to Choose an Indefinite Loop:
- Unknown Iteration Count: Opt for indefinite loops when the number of iterations depends on runtime conditions that are not known in advance. Indefinite loops are adaptable to changing situations.
- Dynamic Control Flow: If your program needs to adapt dynamically to user input, real-time events, or variable response times (e.g., in network programming), indefinite loops are a natural choice.
- Menu-Driven Interfaces: Indefinite loops are commonly used in applications with menu-driven interfaces, where users interact with the program until they decide to exit.
- Handling Uncertain Data Sizes: When dealing with data of uncertain size or when the size can change during execution, such as reading lines from a file or processing user input, indefinite loops are more suitable.
In summary, the choice between a definite loop and an indefinite loop should align with the specific demands of your programming task. Consider the known vs. unknown iteration count, control flow requirements, performance considerations, and the nature of the problem you are solving. In some cases, a combination of both types of loops may be necessary within a larger program to address different aspects of your problem. Ultimately, making the right choice will lead to more efficient and effective code.
FAQs
A definite loop is a type of loop in programming where the number of iterations is known and predetermined in advance. It repeats a set of instructions a fixed number of times.
An indefinite loop is a type of loop in programming where the number of iterations is not known beforehand and depends on a runtime condition. It continues executing as long as the condition remains true.
You should use a definite loop when you have a clear and fixed number of iterations in your task. It provides precise control and is suitable for scenarios where the iteration count is known in advance.
An indefinite loop is appropriate when the number of iterations is uncertain and depends on dynamic conditions. It is commonly used for tasks that adapt to user input, real-time events, or variable response times.
Programming languages like Python, Java, C++, and many others support definite loops, often implemented using “for” loops.
Indefinite loops are typically implemented using “while” loops and are supported by languages like Python, Java, C++, and more.
Definite loops provide predictability, precise control over iteration count, and are often more efficient in terms of performance when the number of iterations is fixed.
Indefinite loops offer flexibility, adaptability to changing conditions, and are suitable for scenarios with unknown iteration counts.
Common pitfalls with definite loops include off-by-one errors in the iteration count, array indexing errors, and using them in situations with uncertain iteration requirements.
Common pitfalls with indefinite loops include creating infinite loops without proper exit conditions, using incorrect or ambiguous exit conditions, and not managing resource consumption effectively.
Read More :
Contents