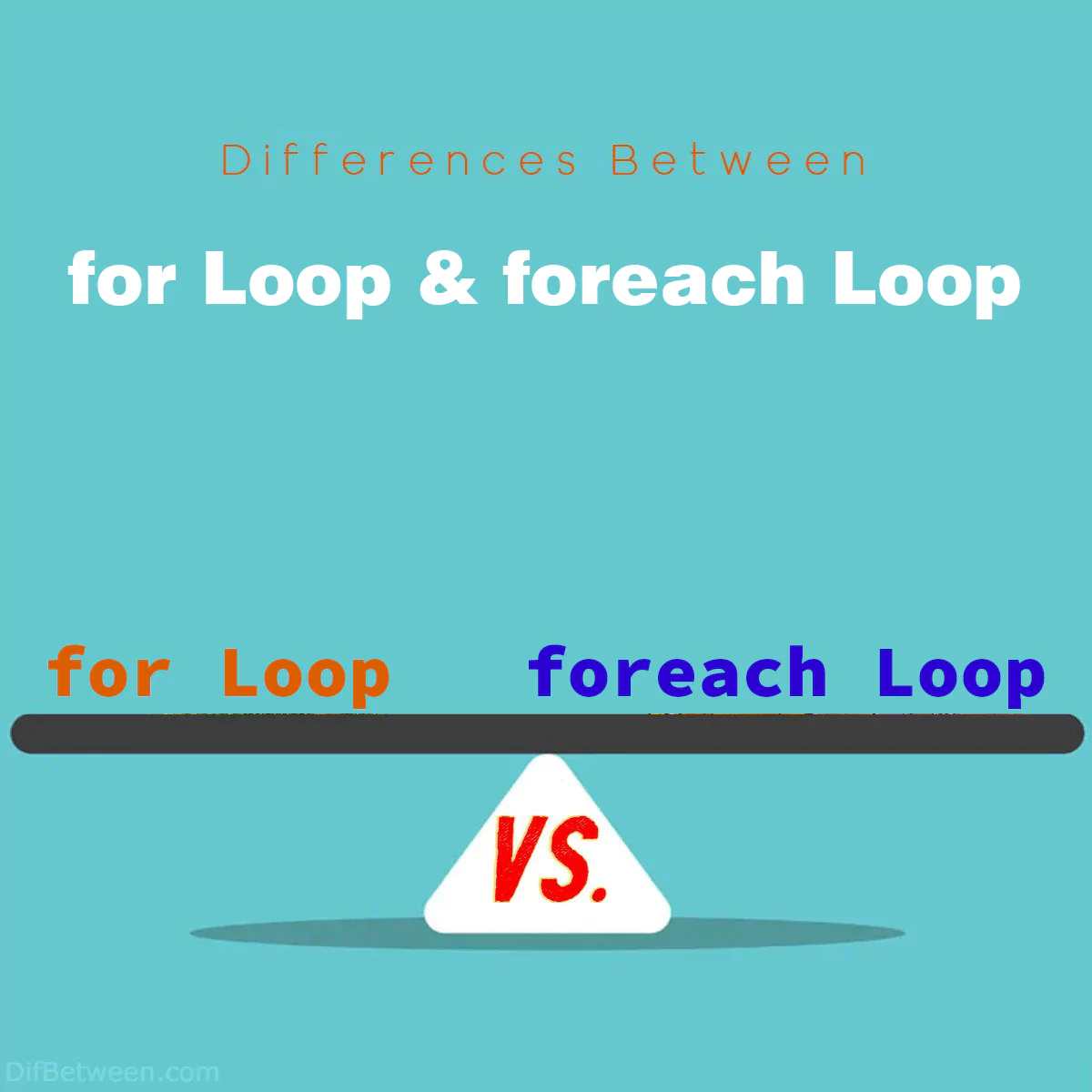
Aspect | “for Loop” | “foreach Loop” |
---|---|---|
Control Mechanism | Explicit control over initialization, condition, and increment/decrement. | Automatic iteration through elements of a collection. |
Syntax | Structured syntax with initialization, condition, and increment/decrement. | Simpler syntax with an item and collection. |
Use Cases | – When you know the exact number of iterations needed. <br>- For fine-grained control over loop variables. <br>- When working with numerical ranges. | – Iterating through collections like arrays, lists, or dictionaries. <br>- Enhancing code readability. <br>- Reducing the risk of off-by-one errors. |
Index Access | Access elements by index. | Access elements directly by their values. |
Collection Modification | Can modify the collection but requires caution. | Generally safer for not modifying the collection. |
Performance | Generally more efficient, especially for large collections. | Slightly less efficient due to additional overhead. |
Compatibility with Data Types | Versatile, can iterate over various data types. | Designed primarily for iterating through collections. |
Readability and Code Length | May result in longer and less concise code. | Results in shorter, cleaner, and more readable code. |
When it comes to programming, loops are a crucial concept that every developer encounters. They allow you to repeat a set of instructions multiple times, making your code more efficient and less repetitive. Two commonly used types of loops are the “for” loop and the “foreach” loop. While they serve a similar purpose, they have distinct differences in terms of their usage and functionality. In this comprehensive guide, we will delve into the key differences between these two loops, shedding light on when and how to use them effectively.
Differences Between for Loop and foreach Loop
The main differences between a “for loop” and a “foreach loop” lie in their control mechanisms and use cases. A “for loop” offers precise control over initialization, conditions, and increments, making it ideal for situations where you know the exact number of iterations or need to manipulate loop variables with precision. On the other hand, a “foreach loop” abstracts away these controls and simplifies code, making it especially useful when iterating through collections like arrays or lists, prioritizing readability, and reducing the risk of off-by-one errors. Ultimately, the choice depends on your specific programming needs, with “for loops” offering fine-grained control, while “foreach loops” emphasize simplicity and readability, particularly in collection-based tasks.
1. Loop Control
The most significant distinction between these two loops lies in how they control the iteration process:
- For Loop: In a “for” loop, you have full control over the initialization, condition, and increment/decrement steps. This level of control makes “for” loops versatile and suitable for situations where you need to iterate a specific number of times or manipulate loop variables precisely.
- Foreach Loop: On the other hand, a “foreach” loop abstracts away the control mechanisms. It automatically iterates through the elements of a collection, providing you with the current element for each iteration. This simplicity reduces the risk of bugs related to index manipulation and makes “foreach” loops more intuitive for iterating over collections.
2. Use Cases
The choice between “for” and “foreach” loops often depends on the specific requirements of your code:
- For Loop Use Cases:
- When you need to iterate a specific number of times.
- For precise control over loop variables.
- When you need to iterate over a range of values.
- Performing mathematical operations with indices.
- Foreach Loop Use Cases:
- When iterating through collections like arrays, lists, or dictionaries.
- Simplifying code for readability when the index is not essential.
- Reducing the risk of off-by-one errors.
3. Index Access
Another crucial difference is how you access elements in the loop:
- For Loop: In a “for” loop, you typically access elements by their index in the collection. This means you can directly manipulate the index to access specific elements or control the loop’s behavior based on the index.
- Foreach Loop: In a “foreach” loop, you access elements directly by their values. This simplifies the code, as you don’t need to manage indices, making it more readable and less error-prone.
Here’s a quick comparison in Python:
For Loop:
fruits = ["apple", "banana", "cherry"] for i in range(len(fruits)): print(fruits[i])
Foreach Loop:
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
In the “for” loop, we explicitly use the index to access elements, while the “foreach” loop directly provides the values of the elements.
4. Collection Modification
When it comes to modifying a collection while iterating through it, the behavior differs:
- For Loop: Using a “for” loop, you can modify the collection as you iterate through it, but you must be cautious. Modifying the collection’s size or structure can lead to unexpected behavior and errors.
- Foreach Loop: A “foreach” loop is generally safer when it comes to modifying a collection. Some programming languages may even throw exceptions if you attempt to modify the collection within a “foreach” loop. It’s a good practice to avoid modifying the collection during iteration with a “foreach” loop.
5. Performance Considerations
Performance is a critical factor to consider when choosing between “for” and “foreach” loops:
- For Loop: “For” loops tend to be more efficient in terms of performance, especially when dealing with large collections. Since you have control over the iteration process, you can optimize it for specific scenarios.
- Foreach Loop: “Foreach” loops are generally less efficient in terms of performance compared to “for” loops. They involve additional overhead because the programming language must manage the iteration process for you. However, the difference in performance is often negligible for most applications unless you’re working with extremely large data sets.
Here’s a simplified performance comparison in C++:
For Loop:
for (int i = 0; i < 1000000; i++) { // Code here }
Foreach Loop (C++11 and later):
std::vector<int> numbers = { /* a million numbers */ }; for (int number : numbers) { // Code here }
In this scenario, the “for” loop is likely to be slightly faster due to its lower overhead.
6. Compatibility with Different Data Types
The compatibility of “for” and “foreach” loops with various data types can also influence your choice:
- For Loop: “For” loops are versatile and can iterate over a wide range of data types, including numerical ranges, arrays, and more. They are not limited to specific data structures.
- Foreach Loop: “Foreach” loops are designed primarily for iterating through collections like arrays, lists, or dictionaries. They are not suitable for iterating over numerical ranges or other non-collection data types.
7. Readability and Code Length
The readability and conciseness of your code are essential considerations:
- For Loop: “For” loops often require more lines of code due to the explicit control mechanisms. This can make your code longer and potentially harder to read, especially for beginners.
- Foreach Loop: “Foreach” loops are generally more concise and expressive. They make your code cleaner and easier to understand, especially when iterating through collections.
for Loop or foreach Loop : Which One is Right Choose for You?
Choosing between a “for” loop and a “foreach” loop depends on the specific requirements of your programming task and your coding preferences. Let’s break down when each type of loop is the right choice:
Use a “for” Loop When:
- Precise Control is Required: If you need fine-grained control over initialization, condition, and increment/decrement, a “for” loop is the better choice. This is especially useful when you know the exact number of iterations needed or when working with numerical ranges.
- Modifying the Collection: When you need to modify the collection while iterating through it, a “for” loop can be used. However, exercise caution to avoid unexpected behavior.
- Numerical Ranges: When your iteration involves numerical ranges or other scenarios where explicit control over loop variables is essential, a “for” loop is more suitable.
- Compatibility with Various Data Types: “For” loops are versatile and can iterate over a wide range of data types, making them suitable for different scenarios beyond collections.
Use a “foreach” Loop When:
- Iterating Through Collections: If your primary task is to iterate through collections like arrays, lists, or dictionaries, a “foreach” loop simplifies the process and enhances code readability.
- Code Readability Matters: When clean and readable code is a priority, “foreach” loops shine. They eliminate the need for explicit index management, reducing the chances of off-by-one errors.
- Minimizing Code Length: “Foreach” loops result in shorter and more concise code compared to “for” loops, making them a good choice for more straightforward and readable code.
- Elements, Not Indices, are the Focus: When you want to focus on the elements themselves rather than their indices, “foreach” loops provide a more natural and intuitive approach.
In summary, the choice between a “for” loop and a “foreach” loop depends on the specific task at hand and your coding priorities. “For” loops offer greater control and versatility, while “foreach” loops excel in readability and simplicity, especially when working with collections. Understanding the strengths and use cases of each type of loop empowers you to write more efficient and maintainable code for your programming projects.
FAQs
A ‘for loop’ is a fundamental programming construct that allows you to execute a block of code repeatedly for a specified number of iterations. It consists of three essential components: initialization, a condition, and an increment (or decrement) operation. These elements work together to control the loop’s behavior, making it suitable for tasks where you need precise control over the iteration process.
You should use a ‘for loop’ when you know the exact number of iterations required or when you need fine-grained control over loop variables. It’s particularly useful for tasks like iterating through numerical ranges, performing mathematical operations with indices, or modifying a collection while iterating.
A ‘foreach loop’ is another programming construct used for iteration, primarily designed for iterating through collections like arrays, lists, or dictionaries. It offers a simpler and more intuitive syntax, abstracting away explicit control mechanisms. Instead of managing indices, you directly access elements, enhancing code readability and reducing the risk of off-by-one errors.
You should use a ‘foreach loop’ when your main task involves iterating through collections, and you want to prioritize code readability and simplicity. It excels in scenarios where you’re working with data structures and want to focus on the elements themselves rather than their indices.
While it’s possible to modify a collection within a ‘for loop,’ it’s generally safer to avoid doing so in a ‘foreach loop.’ Some programming languages may even throw exceptions if you attempt to modify the collection during iteration with a ‘foreach loop.’ If modification is necessary, consider using a ‘for loop’ with caution.
In terms of performance, ‘for loops’ are generally more efficient, especially when dealing with large collections. They have lower overhead because you have explicit control over the iteration process. ‘Foreach loops,’ while slightly less efficient due to additional management by the programming language, are often sufficient for most applications, unless you’re working with extremely large data sets.
No, ‘for loops’ are versatile and can iterate over various data types, including numerical ranges, arrays, and more. They are not limited to specific data structures, making them suitable for a wide range of programming scenarios.
‘Foreach loops’ typically result in shorter and more concise code compared to ‘for loops.’ They simplify code by eliminating the need for explicit index management, making the code cleaner and more readable, particularly when working with collections.
The choice depends on your specific requirements. Use a ‘for loop’ when you need precise control, know the exact number of iterations, or are working with numerical ranges. Opt for a ‘foreach loop’ when iterating through collections, prioritizing code readability, and minimizing the risk of off-by-one errors.
Yes, you can nest ‘for loops’ within other ‘for loops’ and ‘foreach loops’ within other ‘foreach loops’ or ‘for loops.’ This allows you to handle more complex iteration scenarios, such as multi-dimensional arrays or nested data structures, by controlling different levels of iteration simultaneously. However, it’s essential to manage variables and conditions carefully to avoid unintended behavior and infinite loops.
Read More :
Contents