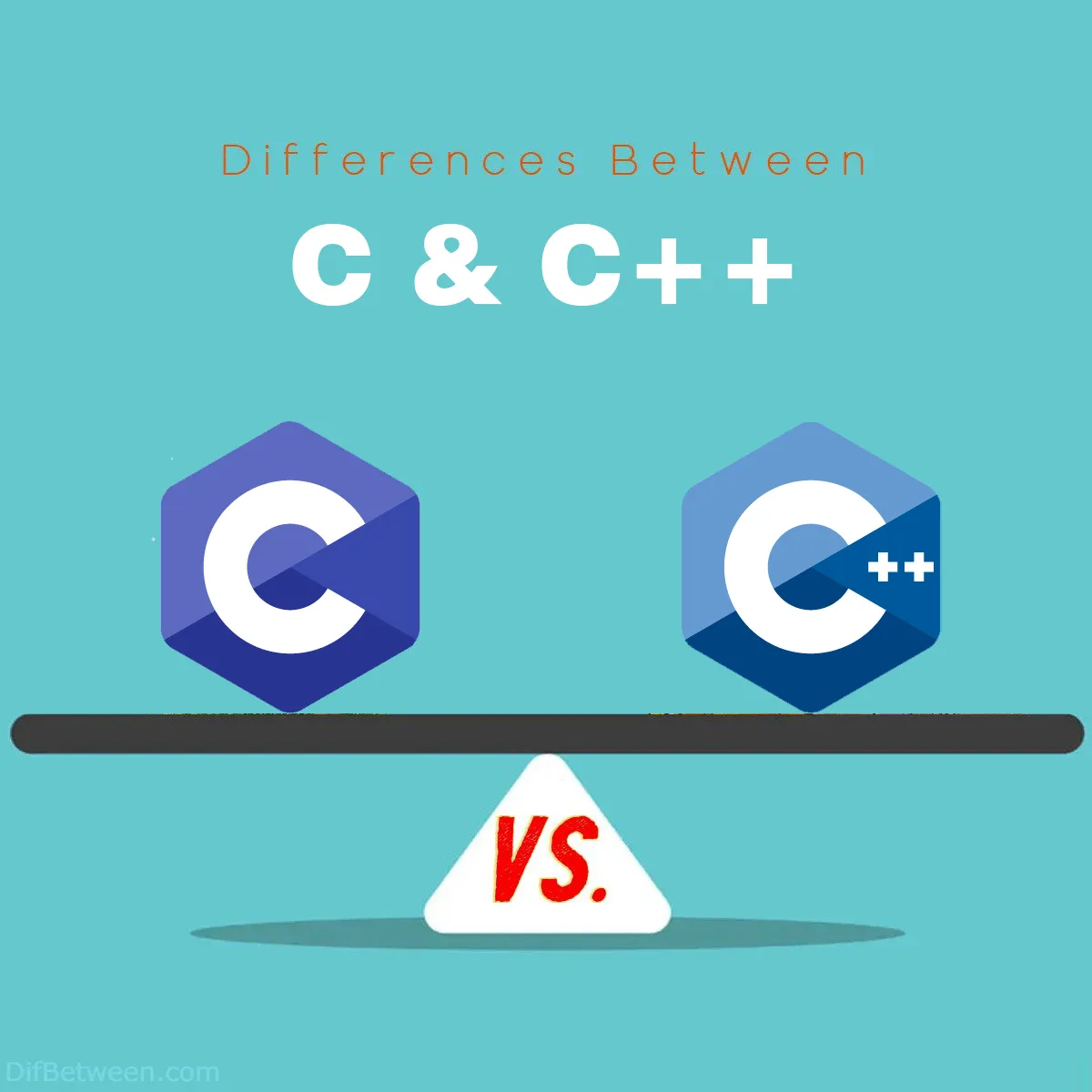
Aspect | C | C++ |
---|---|---|
Programming Paradigm | Procedural/Structured | Procedural and Object-Oriented |
Code Structure | Modular (Functions and Files) | Classes and Objects, Modular |
Memory Management | Manual (malloc(), free()) | Automatic (RAII, smart pointers) |
Standard Library | Minimalist (libc) | Feature-rich (STL, iostream, etc.) |
Error Handling | Return Values and Global Variables | Exceptions (try-catch) |
Function Overloading and Default Args | No Function Overloading, No Default Args | Function Overloading, Default Args |
Object-Oriented Programming (OOP) | Limited or Manual Implementation | Full Support for OOP |
Multiple Inheritance | Not Supported | Supported (with some limitations) |
Operator Overloading | Not Supported | Supported |
Standard Revisions | C89, C99, C11, C18 | C++98, C++11, C++14, C++17, C++20, C++23 |
Backward Compatibility | Strong Emphasis | Emphasis (with occasional changes) |
In the world of programming, C and C++ are like two siblings sharing a common ancestry. Both languages are renowned for their power and flexibility, making them essential tools for developers worldwide. However, despite their similarities, there are some distinct differences that set them apart. In this comprehensive guide, we’ll dive into the key differences between C and C++ to help you understand when and why to choose one over the other.
Differences Between C and C++
The main differences between C and C++ revolve around their programming paradigms and feature sets. C is a procedural language, primarily focused on functions and structured programming, with manual memory management and a minimalistic standard library (libc). In contrast, C++ extends C by introducing object-oriented programming (OOP) capabilities, automatic memory management through RAII, a feature-rich Standard Template Library (STL), and support for modern programming practices. While C emphasizes simplicity, portability, and backward compatibility, C++ excels in complex software development, offering a versatile toolset for efficient, object-oriented, and feature-rich programming.
1. C vs. C++: The Fundamental Difference
Let’s start with the most fundamental difference between C and C++: their core philosophy. C is often described as a “procedural” or “structured” programming language. It was designed with a focus on functions and procedures, making it an excellent choice for tasks where control over low-level hardware is crucial. C++, on the other hand, extends C by introducing object-oriented programming (OOP) features. This means that C++ not only supports the procedural style but also allows you to use OOP concepts like classes and objects.
C: Procedural Programming
In C, the main approach to problem-solving is through procedures or functions. You break down your program into a series of functions, each responsible for a specific task. These functions can be called in a sequence to achieve the desired outcome. While C doesn’t have built-in support for concepts like classes or objects, you can still use structs to organize related data.
Procedural programming in C is like building a complex machine with various interconnected gears and levers. Each function is a cog in the machine, and they work together to achieve the desired result. This approach is efficient and works well for tasks where you need precise control over memory and hardware resources.
C++: Object-Oriented Programming (OOP)
C++ takes a different approach by introducing the world of object-oriented programming. In OOP, you model real-world entities as objects, each encapsulating both data and the functions that operate on that data. This paradigm provides a more intuitive way to design and structure your code, as it aligns with the natural organization of real-world concepts.
Consider a simple example: a program that manages shapes. In C++, you can define a Shape
class with data members (attributes) like the shape’s dimensions and member functions (methods) to calculate its area or perimeter. You can then create instances of this class, such as Circle
and Rectangle
, which inherit the common properties and methods from the Shape
class.
In summary, the fundamental difference lies in the programming paradigms: C is primarily procedural, while C++ offers the versatility of both procedural and object-oriented programming.
2. C vs. C++: Code Structure
The way you structure your code in C and C++ can also vary significantly. Let’s explore how they differ in terms of code organization and modularity.
C: Modular Programming
C encourages modular programming, where you divide your code into separate functions and files. Each function has a specific responsibility, and you can group related functions into header (.h) and source (.c) files. This separation of concerns allows for better code organization and reuse.
C programs typically consist of multiple source files, each containing one or more functions. You use header files to declare function prototypes and include them in the source files that need those functions. This modular approach makes it easier to manage large codebases and promotes code reusability.
Here’s a simple example of modular C code:
// math_operations.h #ifndef MATH_OPERATIONS_H #define MATH_OPERATIONS_H int add(int a, int b); int subtract(int a, int b); #endif
// math_operations.c #include "math_operations.h" int add(int a, int b) { return a + b; } int subtract(int a, int b) { return a - b; }
C++: Classes and Objects
In C++, the use of classes and objects is a central part of code organization. You define classes to encapsulate data and behavior related to a specific entity. These classes are typically declared in header files (.h) and implemented in source files (.cpp).
C++’s approach to code structure is object-oriented, and it encourages you to think in terms of objects and their interactions. For example, if you are developing a game, you might have classes for characters, weapons, and levels, each with its own data members and member functions.
Here’s a simple example of C++ code with classes:
// Circle.h #ifndef CIRCLE_H #define CIRCLE_H class Circle { private: double radius; public: Circle(double r); double calculateArea(); }; #endif
// Circle.cpp #include "Circle.h" #include <cmath> Circle::Circle(double r) { radius = r; } double Circle::calculateArea() { return 3.14159265358979323846 * std::pow(radius, 2); }
In summary, C promotes modular programming with functions and files, while C++ emphasizes the use of classes and objects for code organization.
3. Memory Management
Memory management is a critical aspect of programming, and C and C++ handle it differently. Let’s explore how they approach memory management.
C: Manual Memory Management
In C, you have complete control over memory management. You allocate and deallocate memory explicitly using functions like malloc()
and free()
. This manual approach gives you fine-grained control but also comes with the responsibility of ensuring that you release allocated memory to prevent memory leaks.
Here’s an example of dynamic memory allocation in C:
#include <stdio.h> #include <stdlib.h> int main() { int *arr = (int *)malloc(5 * sizeof(int)); // Allocate memory for an integer array if (arr == NULL) { printf("Memory allocation failed.\n"); return 1; } // Use the allocated memory for (int i = 0; i < 5; i++) { arr[i] = i * 2; } // Deallocate the memory free(arr); return 0; }
While manual memory management in C provides control, it can be error-prone, leading to memory leaks or segmentation faults if not done correctly.
C++: Automatic Memory Management (RAII)
C++ simplifies memory management through a concept called Resource Acquisition Is Initialization (RAII). RAII ties the lifetime of resources, such as memory allocations, to the lifespan of objects. When an object goes out of scope, its destructor is automatically called, allowing for resource cleanup.
C++ achieves this by introducing features like constructors and destructors. When you create an object, its constructor is called to allocate any necessary resources. When the object goes out of scope, its destructor is called to release those resources. This automatic management reduces the risk of memory leaks.
Here’s a C++ example using RAII for dynamic memory management:
#include <iostream> #include <memory> int main() { std::unique_ptr<int[]> arr = std::make_unique<int[]>(5); // Allocate memory for an integer array // Use the allocated memory for (int i = 0; i < 5; i++) { arr[i] = i * 2; } // No need to deallocate explicitly; memory is automatically released when arr goes out of scope return 0; }
In summary, C requires manual memory management using functions like malloc()
and free()
, while C++ provides automatic memory management through RAII, reducing the risk of memory-related errors.
4. Standard Libraries
Both C and C++ have their own standard libraries that provide a set of functions and classes for common tasks. However, there are differences in how these libraries are structured and what they offer.
C: Standard C Library (libc)
The Standard C Library, often referred to as libc
, is a minimalist library that provides fundamental functions for input/output, string manipulation, memory management, and mathematical operations. It is designed to be small and efficient, reflecting C’s philosophy of “no overhead unless explicitly requested.”
Here are some common functions from the C Standard Library:
printf()
andscanf()
for input/outputstrcpy()
andstrcat()
for string manipulationmalloc()
andfree()
for memory managementsqrt()
andsin()
for mathematical operations
C’s libc
is known for its simplicity and portability. It provides a solid foundation for building various applications, from embedded systems to high-performance software.
C++: Standard Template Library (STL)
C++ introduces the Standard Template Library (STL), which is a collection of template classes and functions. The STL is a powerful library that offers a wide range of data structures (e.g., vectors, lists, maps) and algorithms (e.g., sorting, searching) that are highly reusable and customizable.
The key feature of the STL is its use of templates, which allows you to create generic data structures and algorithms that work with different data types. This makes the STL highly adaptable and promotes code reusability.
Here are some components of the C++ Standard Template Library:
std::vector
for dynamic arraysstd::map
for associative arrays (key-value pairs)std::sort()
andstd::search()
for sorting and searching algorithmsstd::string
for string manipulation
The STL is an integral part of C++ and aligns with the language’s emphasis on abstraction and object-oriented design.
In summary, C provides the minimalist libc
for basic functionality, while C++ offers the feature-rich STL with templates for creating reusable data structures and algorithms.
5. Error Handling
Error handling is a crucial aspect of software development. Both C and C++ provide mechanisms for handling errors, but they differ in their approaches.
C: Error Handling with Return Values
In C, error handling is typically done using return values. Functions often return a special value (e.g., -1) or set a global variable (e.g., errno
) to indicate errors. The calling code is responsible for checking these values and taking appropriate action.
Here’s an example of error handling in C:
#include <stdio.h> int divide(int a, int b, int *result) { if (b == 0) { // Division by zero error return -1; } *result = a / b; return 0; // Success } int main() { int a = 10, b = 0, result; if (divide(a, b, &result) == -1) { printf("Error: Division by zero.\n"); } else { printf("Result: %d\n", result); } return 0; }
In this example, the divide()
function returns -1 to indicate an error when dividing by zero.
C++: Exception Handling
C++ introduces a more robust mechanism for error handling called exception handling. Instead of returning error codes, functions in C++ can throw exceptions when errors occur. The calling code can then catch and handle these exceptions using try
and catch
blocks.
Here’s an example of exception handling in C++:
#include <iostream> int divide(int a, int b) { if (b == 0) { throw std::runtime_error("Division by zero error"); } return a / b; } int main() { int a = 10, b = 0; try { int result = divide(a, b); std::cout << "Result: " << result << std::endl; } catch (const std::runtime_error& e) { std::cerr << "Error: " << e.what() << std::endl; } return 0; }
In this C++ example, the divide()
function throws an exception when dividing by zero, and the try
block in main()
catches the exception, allowing for more graceful error handling.
In summary, C relies on return values and global variables for error handling, while C++ provides a more structured approach using exceptions and try
/catch
blocks.
6. Function Overloading and Default Arguments
C++ introduces some powerful features related to function definitions and calls that are not available in C. These features provide greater flexibility and code readability.
C: Single Function Definition
In C, if you want to create multiple functions with the same name but different parameter lists (function overloading), you need to define distinct names for each function. This can lead to naming complexity and reduced code clarity.
Here’s an example of function overloading in C:
#include <stdio.h> int add_int(int a, int b) { return a + b; } float add_float(float a, float b) { return a + b; } int main() { int sum_int = add_int(2, 3); float sum_float = add_float(2.5, 3.7); printf("Integer sum: %d\n", sum_int); printf("Float sum: %.2f\n", sum_float); return 0; }
In C, you must give each overloaded function a unique name (add_int
and add_float
in this case).
C++: Function Overloading and Default Arguments
C++ simplifies function overloading by allowing you to define multiple functions with the same name, differing only in the number or type of parameters. This enhances code readability and reduces naming complexity.
Here’s an example of function overloading in C++:
#include <iostream> int add(int a, int b) { return a + b; } double add(double a, double b) { return a + b; } int main() { int sum_int = add(2, 3); double sum_double = add(2.5, 3.7); std::cout << "Integer sum: " << sum_int << std::endl; std::cout << "Double sum: " << sum_double << std::endl; return 0; }
In C++, you can have functions with the same name (add
in this case), differing in the types of their parameters.
Additionally, C++ allows you to specify default argument values for function parameters. This means you can define functions with optional parameters that have default values, simplifying function calls and reducing the need for overloaded functions.
Here’s an example of default arguments in C++:
#include <iostream> int multiply(int a, int b = 2) { return a * b; } int main() { int result1 = multiply(5); // Uses default value for b int result2 = multiply(5, 3); // Overrides default value std::cout << "Result 1: " << result1 << std::endl; std::cout << "Result 2: " << result2 << std::endl; return 0; }
In this C++ example, the multiply
function has a default argument for b
, so you can call it with one or two arguments.
In summary, C++ offers function overloading and default argument values, making code more concise and expressive compared to C.
7. Object-Oriented Programming (OOP) Features
As mentioned earlier, C++ extends C by introducing powerful object-oriented programming (OOP) features. Let’s explore some of these features in more detail.
C: No Built-in OOP Support
C lacks built-in support for object-oriented programming. While you can use structs to group related data members, there are no built-in concepts like classes, objects, inheritance, or polymorphism. You must manually implement OOP principles if needed, which can be complex and error-prone.
Here’s an example of a C struct used for data encapsulation:
#include <stdio.h> struct Circle { double radius; }; double calculateArea(struct Circle c) { return 3.14159265358979323846 * c.radius * c.radius; } int main() { struct Circle myCircle; myCircle.radius = 5.0; double area = calculateArea(myCircle); printf("Circle area: %.2f\n", area); return 0; }
In this C example, a struct is used to encapsulate the data related to a circle.
C++: Full OOP Support
C++ provides comprehensive support for object-oriented programming. It introduces classes and objects, enabling you to create blueprints for objects and instantiate them. You can also implement concepts like inheritance, polymorphism, and encapsulation.
Here’s a C++ example that uses a class to represent a circle:
#include <iostream> class Circle { private: double radius; public: Circle(double r) : radius(r) {} double calculateArea() { return 3.14159265358979323846 * radius * radius; } }; int main() { Circle myCircle(5.0); double area = myCircle.calculateArea(); std::cout << "Circle area: " << area << std::endl; return 0; }
In this C++ example, a Circle
class is defined with data members (radius) and a member function (calculateArea), demonstrating the use of encapsulation.
In summary, C++ brings robust support for object-oriented programming, while C relies on manual techniques to achieve similar results.
8. Multiple Inheritance
Multiple inheritance is a feature that allows a class to inherit attributes and behaviors from more than one base class. This feature is supported in C++ but not in C.
C++: Multiple Inheritance
In C++, you can create a class that inherits from multiple base classes. This allows you to combine features from different classes into a single derived class. Multiple inheritance can be a powerful tool for code reuse and modeling complex relationships.
Here’s an example of multiple inheritance in C++:
#include <iostream> class Shape { public: void display() { std::cout << "Shape" << std::endl; } }; class Color { public: void display() { std::cout << "Color" << std::endl; } }; class ColoredShape : public Shape, public Color { public: void displayBoth() { Shape::display(); Color::display(); } }; int main() { ColoredShape cs; cs.displayBoth(); return 0; }
In this C++ example, the ColoredShape
class inherits from both Shape
and Color
, and it can access members from both base classes.
C: No Multiple Inheritance
C does not support multiple inheritance. In C, you can only inherit from a single base structure, limiting your ability to combine features from different sources directly. If you need to achieve similar results in C, you would typically use structures or pointers to structures to represent complex relationships.
In summary, multiple inheritance is a feature available in C++ that allows classes to inherit from multiple base classes, while C does not support this feature.
9. Operator Overloading
Operator overloading is a feature that allows you to define custom behaviors for operators when applied to user-defined types. This feature is available in C++ but not in C.
C++: Operator Overloading
C++ enables you to overload operators, allowing you to define how operators work with your custom data types. This feature can make your code more expressive and intuitive by providing natural syntax for user-defined classes.
Here’s an example of operator overloading in C++:
#include <iostream> class Complex { private: double real; double imaginary; public: Complex(double r, double i) : real(r), imaginary(i) {} Complex operator+(const Complex& other) { return Complex(real + other.real, imaginary + other.imaginary); } void display() { std::cout << real << " + " << imaginary << "i" << std::endl; } }; int main() { Complex c1(2.0, 3.0); Complex c2(1.0, 2.0); Complex sum = c1 + c2; sum.display(); return 0; }
In this C++ example, the +
operator is overloaded for the Complex
class to perform addition of complex numbers in an intuitive way.
C: No Operator Overloading
C does not support operator overloading. In C, operators have fixed meanings for built-in data types, and you cannot redefine their behavior for user-defined types.
In summary, operator overloading is a feature available in C++ that allows you to redefine the behavior of operators for user-defined types, while C does not offer this capability.
C or C++ : Which One is Right To Choose?
The choice between C and C++ depends on your specific project requirements, your team’s expertise, and the trade-offs you are willing to make. Let’s examine scenarios in which each language might be the right choice:
Choose C if:
- Portability and Compatibility: If you need a high degree of portability and compatibility across different systems and platforms, C is an excellent choice. Code written in C tends to be more portable because it relies on a minimalist standard library and has a strong emphasis on backward compatibility.
- Low-Level Programming: For tasks that require low-level control over hardware resources, such as embedded systems programming, device drivers, or operating systems development, C is often the preferred language due to its simplicity and predictable behavior.
- Legacy Codebase: If your project involves maintaining or extending a legacy codebase that was written in C, it makes sense to continue using C for consistency and ease of integration with existing code.
- Small Codebase: For smaller projects or scripts where the overhead of object-oriented programming and the Standard Template Library (STL) might not be justified, C offers a lightweight option.
- Efficiency and Performance: When optimizing for efficiency and performance, C allows you to have precise control over memory management and minimize runtime overhead.
Choose C++ if:
- Object-Oriented Design: If you are developing software with complex relationships and require an object-oriented design, C++ is the natural choice. It provides support for classes, objects, inheritance, and polymorphism, making it easier to model real-world entities.
- Rich Standard Library: C++ offers a feature-rich Standard Template Library (STL) that includes data structures, algorithms, and utilities, saving you development time and effort. If you need to work with complex data structures or perform high-level operations, C++ can be more productive.
- Modern Programming Practices: C++ standards (e.g., C++11 and beyond) introduce modern programming features and enhancements, such as lambda expressions, smart pointers, and improved type safety. If you want to leverage these features, C++ is the way to go.
- Compatibility with Existing C Libraries: C++ can seamlessly integrate with existing C libraries, allowing you to benefit from C’s extensive ecosystem while also incorporating object-oriented features into your project.
- Large and Complex Projects: For large and complex software projects, C++’s support for encapsulation, modularity, and abstraction can lead to more maintainable and scalable codebases.
- Safety and Abstraction: If safety and code abstraction are critical, C++’s exception handling and automatic resource management (RAII) can help you write safer and more reliable code.
In many cases, the choice may not be binary, and you can leverage both languages in a single project. Some projects use C for low-level components and C++ for higher-level application logic, taking advantage of the strengths of both languages.
Ultimately, the decision should align with your project’s goals and constraints. Consider factors such as your team’s expertise, the existing codebase, performance requirements, and the long-term maintainability of the project when making your choice between C and C++.
FAQs
The main difference is in their programming paradigms. C is primarily a procedural language, while C++ extends C with support for both procedural and object-oriented programming (OOP). C++ introduces features like classes, objects, inheritance, and polymorphism.
You might choose C when you need low-level control over hardware resources, work with legacy codebases, or require high portability and compatibility. C is also a good choice for small projects and situations where the overhead of OOP is not justified.
C++ is a better choice for projects that benefit from OOP principles, rich libraries (STL), and modern programming features. It’s suitable for large and complex software development, where encapsulation and abstraction are important. Additionally, C++ integrates well with existing C libraries.
Both C and C++ offer similar performance since they compile to machine code. The efficiency depends more on how you write your code rather than the language itself. However, C++ can be more efficient in terms of developer productivity due to its higher-level abstractions.
Yes, you can use C libraries in C++ seamlessly. C++ is designed to be compatible with C, and you can include and call C functions and use C headers without any issues.
Some advantages of C++ over C include better support for OOP, automatic memory management (RAII), a rich standard library (STL), and modern programming features like lambda expressions and smart pointers.
Yes, C is still relevant in various domains, such as embedded systems, operating systems, and low-level programming. It provides control and portability that are essential in these areas.
C++ can be seen as more beginner-friendly due to its higher-level abstractions and support for OOP. However, both languages are accessible to beginners with the right resources and guidance.
Converting C code to C++ is generally possible, but it may require some adjustments, especially if you want to leverage C++’s OOP features. The process depends on the complexity of the code and your specific goals.
Yes, it’s possible to mix C and C++ code in the same project. C++ was designed to be compatible with C, so you can gradually transition from one language to the other if needed. This is commonly done in practice to take advantage of both languages’ strengths.
Read More :