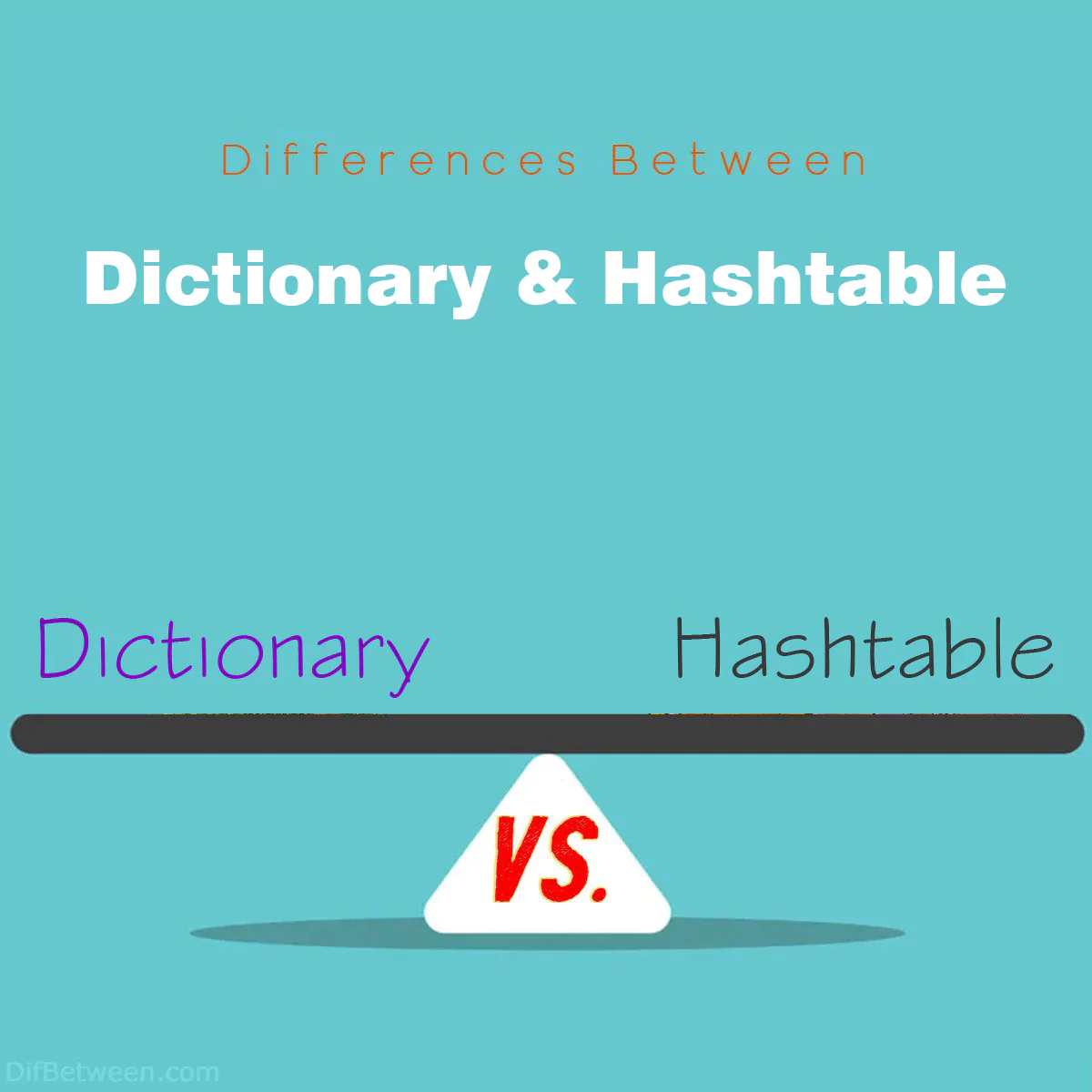
Aspect | Dictionary (Python) | Hashtable (Various Languages) |
---|---|---|
Data Structure | Implemented as hash tables; higher-level interface. Unordered. Mutable. | General term for a data structure using hash functions. Unordered. Mutable. |
Programming Languages | Python, Ruby, JavaScript, Perl, and others. | Found in various programming languages with varying implementations. |
Key Type Restrictions | Keys must be immutable (e.g., strings, numbers, tuples). | Key types and restrictions vary by language; custom key types possible in some languages. |
Null Values | Keys cannot be None or mutable objects. Values can be None . | Handling of null or None values varies by language. |
Collision Resolution | Linear probing (open addressing) for collisions. Potential clustering. | Varies by implementation; techniques like chaining, linear probing, or open addressing. |
Performance | Average O(1) time complexity for retrieval. Can be O(n) in rare cases. | Average O(1) time complexity for retrieval, but it may degrade with collisions. |
Thread Safety | Not thread-safe. | Varies by implementation; may require external synchronization in concurrent environments. |
Memory Usage | Can consume more memory with low fill factor. Dynamically resized. | Memory usage depends on implementation and usage patterns. |
Use Cases | Versatile; used for various purposes, including configuration settings, caching, counting, associative arrays, and lookup tables. | Widely used for key-value pair storage in many software applications. |
In the vast realm of programming, dictionaries and hash tables (often referred to as hashtables) are two essential data structures that hold a special place. These structures are pivotal in storing and retrieving key-value pairs, making them indispensable tools for developers. But what sets them apart? What are the nuances that differentiate a dictionary from a hashtable? Buckle up as we embark on a journey to explore these key differences between dictionary and hashtable.
Differences Between Dictionary and Hashtable
The main differences between a dictionary and a hashtable lie in their implementation and language compatibility. While a dictionary, as commonly found in Python, offers a user-friendly, high-level interface for storing key-value pairs, hashtables are a more general concept used across various programming languages, each with its own implementation details. Dictionaries are known for their simplicity, mutability, and versatility, making them ideal for everyday use cases. In contrast, hashtables are language-agnostic, accommodating custom key types and offering more control over null values and collision resolution strategies, making them suitable for broader, cross-language applications.
1. Data Structure
Dictionary:
At the heart of Python lies the humble dictionary. It’s a built-in data structure used to store collections of data in the form of key-value pairs. In Python, dictionaries are implemented as hash tables, but they offer a higher-level interface, making them more user-friendly.
The primary characteristics of a dictionary include:
- Unordered: Dictionaries in Python are unordered, which means there’s no guaranteed order among the elements. This is because dictionaries use a hash function to organize and access data quickly.
- Mutable: You can modify the contents of a dictionary after it’s created. This includes adding, updating, or removing key-value pairs.
Hashtable:
A hashtable, on the other hand, is a more general term used to describe a data structure that uses a hash function to map keys to values. Unlike Python dictionaries, hashtables are found in various programming languages and libraries, each with its own implementation details.
Hashtables typically exhibit the following characteristics:
- Unordered: Similar to dictionaries, hashtables are also unordered. The order in which elements are stored may not correspond to the order in which they were added.
- Mutable: In many programming languages, including Java, C++, and C#, hashtables are mutable, allowing you to add, update, or remove elements.
2. Programming Languages
Dictionary:
Dictionaries are closely associated with specific programming languages. For example, they are a core data type in Python and Ruby, and they have counterparts in languages like JavaScript (objects) and Perl (hashes).
Let’s take a closer look at Python dictionaries:
Language | Dictionary Representation |
---|---|
Python | my_dict = {'key1': 'value1', 'key2': 'value2'} |
JavaScript | let myObj = {key1: 'value1', key2: 'value2'}; |
Ruby | my_hash = {'key1' => 'value1', 'key2' => 'value2'} |
Perl | %my_hash = ('key1' => 'value1', 'key2' => 'value2'); |
Hashtable:
Hashtables, on the other hand, are not tied to specific programming languages. They are a fundamental concept in computer science and can be implemented in various languages. Some programming languages have built-in support for hashtables, while others require the use of libraries or custom implementations.
Here’s how hashtables are represented in a few programming languages:
Language | Hashtable Representation |
---|---|
Java | HashMap<String, String> myMap = new HashMap<>(); |
C# | Dictionary<string, string> myDict = new Dictionary<>(); |
C++ | std::unordered_map<std::string, std::string> myMap; |
JavaScript | const myMap = new Map(); |
3. Key Type Restrictions
Dictionary:
In Python dictionaries, the keys are subject to certain restrictions:
- Immutable Keys: Dictionary keys must be of an immutable data type, such as strings, numbers, or tuples. This is because the key’s hash value should remain constant.
Here’s an example of a dictionary with various valid key types:
my_dict = {1: 'one', 'two': 2, (3, 4): 'tuple'}
Hashtable:
Hashtables in other programming languages might have different rules regarding key types. In languages like Java and C#, keys are required to be objects that override the hashCode()
and equals()
methods. This allows for custom key types.
Here’s an example of a Java Hashtable with a custom key type:
class CustomKey { private String value; // Constructors, getters, setters, and hashCode() and equals() methods } Hashtable<CustomKey, String> customHashtable = new Hashtable<>();
4. Null Values
Dictionary:
In Python dictionaries, keys cannot be None
or any other mutable object. However, values in a dictionary can be None
. Attempting to use None
as a key will result in a TypeError
.
Example:
my_dict = {None: 'value'} # This will raise a TypeError
Hashtable:
The handling of null
or None
values in hashtables varies depending on the programming language. In languages like Java, keys and values can be null
. In languages like C#, keys cannot be null
, but values can be.
It’s important to consult the documentation of the specific hashtable implementation in your chosen language for details on null value handling.
5. Collision Resolution
Dictionary:
Python dictionaries handle collisions internally using an open addressing technique called linear probing. When two keys have the same hash value, the second key is stored in the next available slot. This process continues until an empty slot is found. Linear probing can potentially lead to clustering, affecting performance.
6. Performance Considerations
Dictionary:
Python dictionaries are highly optimized for typical use cases. Retrieving a value by its key has an average time complexity of O(1). However, in rare cases with hash collisions or when the dictionary needs to be resized, the time complexity can be O(n), where n is the number of key-value pairs.
7. Thread Safety
Dictionary:
In Python, standard dictionaries (i.e., built-in dictionaries) are not thread-safe. If you need thread safety, you should use the threading
or multiprocessing
modules to manage access to dictionaries in a multi-threaded or multi-process environment.
8. Memory Usage
Dictionary:
Python dictionaries are known to consume more memory compared to some other data structures, especially when they have a low fill factor (a high percentage of empty slots). This is because dictionaries are dynamically resized to maintain a balance between load factor and performance.
9. Use Cases
Dictionary:
Dictionaries in Python are versatile and widely used for various purposes, including:
- Storing configuration settings.
- Caching results to optimize performance.
- Counting occurrences of elements in a collection.
- Implementing associative arrays.
- Building simple lookup tables.
Dictionary or Hashtable : Which One is Right To Choose?
Choosing between a dictionary and a hashtable depends on several factors, including the programming language you are using, your specific use case, and your project’s requirements. Let’s break down the decision-making process to help you determine which one is right for your needs:
When to Choose a Dictionary:
- Python: If you are working with Python, dictionaries are the built-in data structure for storing key-value pairs. They are easy to use and well-integrated into the language.
- If you need a simple and straightforward way to store and retrieve key-value pairs in a language that supports dictionaries, they are an excellent choice. Dictionaries are easy to understand and use.
- Dictionaries are versatile and can be used for various purposes, such as storing configuration settings, caching results, counting occurrences, implementing associative arrays, and building simple lookup tables.
- If you require a data structure that allows you to add, update, or remove key-value pairs after creation, dictionaries in languages like Python fit the bill.
- For most everyday use cases where performance is not a critical concern, dictionaries are a suitable choice. Retrieval of values by their keys has an average time complexity of O(1).
- Keep in mind that dictionaries, especially in Python, may consume more memory, especially when they have a low fill factor (a high percentage of empty slots). This dynamic resizing is done to maintain a balance between load factor and performance.
- In Python, keys cannot be
None
or mutable objects, but values can beNone
. If this restriction aligns with your use case, dictionaries may be suitable.
When to Choose a Hashtable:
- Hashtables are not tied to specific programming languages and can be implemented in various languages. If you need a data structure that can be used across different languages, a hashtable might be a better choice.
- Some programming languages, like Java and C#, support hashtables with custom key types. If you need to use non-standard key types or complex objects as keys, hashtables can accommodate this.
- Depending on the language, hashtables may offer more flexibility in handling
null
orNone
values for keys and values. - Hashtables provide various collision resolution strategies, such as chaining, linear probing, or open addressing. If you have specific requirements for handling collisions, you can choose a hashtable implementation that suits your needs.
- While standard dictionaries in some languages are not thread-safe, some hashtable implementations offer built-in thread safety features. If your project requires concurrent access to the data structure, you can consider thread-safe hashtables.
- Hashtables may offer more control over memory usage, depending on the implementation and usage patterns. If memory efficiency is a critical concern, you can optimize the hashtable accordingly.
- If you are dealing with high-performance applications where fine-tuning performance is crucial, you can explore different hashtable implementations and strategies to optimize for your specific use case.
In summary, the choice between a dictionary and a hashtable depends on factors such as language compatibility, simplicity, mutability, performance requirements, memory usage, and specific use case constraints. If your programming language supports dictionaries and your use case aligns with their characteristics, they are often the simplest and most convenient choice. However, if you require features not provided by standard dictionaries, need language-agnostic data structures, or have specific performance or concurrency requirements, exploring hashtable implementations may be the way to go. Ultimately, the decision should be guided by a careful assessment of your project’s unique needs.
FAQs
The main difference lies in their implementation and language compatibility. A dictionary, commonly found in languages like Python, is a high-level interface for storing key-value pairs. In contrast, a hashtable is a more general concept used across various programming languages, each with its own implementation details.
Both dictionaries and hashtables are mutable, allowing you to add, update, or remove key-value pairs after creation. This mutability makes them versatile for dynamic data storage.
In dictionaries (e.g., Python dictionaries), keys must be of immutable types like strings, numbers, or tuples. Hashtables may have different key type requirements depending on the programming language, with some languages allowing custom key types.
In Python dictionaries, keys cannot be None
or mutable objects, but values can be None
. Handling of null
or None
values in hashtables varies by language, with some languages offering more flexibility.
Python dictionaries use linear probing (open addressing) for collision resolution. Hashtables employ various collision resolution strategies depending on the implementation, including chaining, linear probing, or open addressing.
Standard dictionaries in some languages (e.g., Python) are not thread-safe. Whether hashtables are thread-safe depends on the specific implementation; some offer built-in thread safety features, while others may require external synchronization.
he choice depends on factors like language compatibility, simplicity, mutability, performance, memory usage, and your project’s constraints. If you are working within a language that supports dictionaries and your use case aligns with their characteristics, they provide a straightforward solution. Hashtables offer more versatility, cross-language compatibility, and control for specialized needs. Your decision should be based on the specific demands of your project.
Read More :
Contents