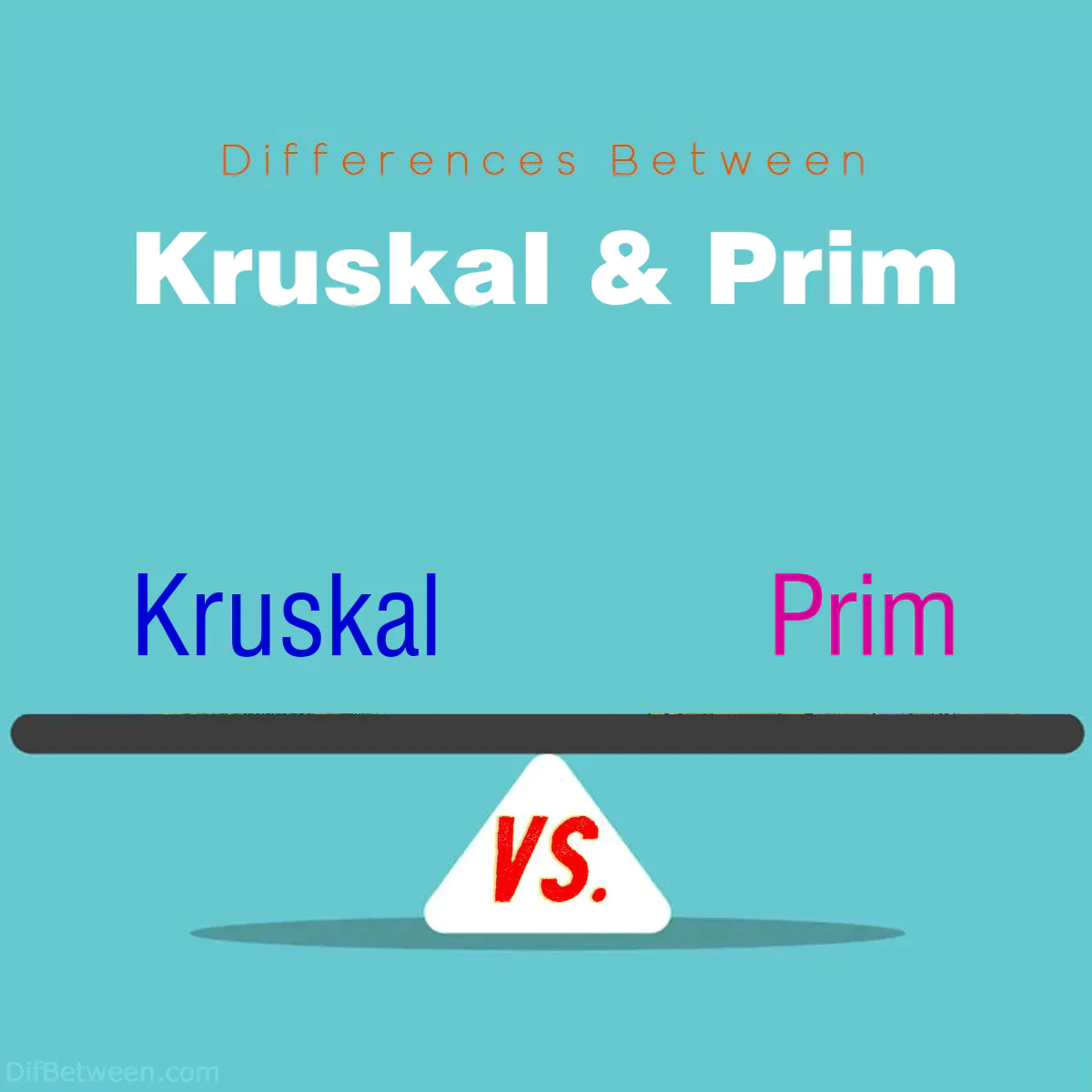
Aspect | Kruskal | Prim |
---|---|---|
Algorithm Approach | Greedy, edge-centric | Greedy, vertex-centric |
Edge Selection Criteria | Based solely on weight | Based on connection to the tree |
Time Complexity | O(E log E) | O(V^2) or O(E log V) |
Space Complexity | O(V + E) | O(V) |
Suitable Graph Types | Sparse graphs, various edge weights | Dense graphs, highly connected trees |
Parallelization Potential | Highly parallelizable during sorting | Less straightforward for parallelization |
Handling Negative Weights | Handles negative weights naturally | May require modifications for negatives |
Applications | Network design, clustering, chemistry | Networking, robotics, circuit design |
Handling Disconnected Components | Finds minimum spanning forest | Assumes a connected graph |
Implementation Complexity | Relatively straightforward | Requires careful management of connectivity |
Robustness to Weight Perturbations | Robust to small weight changes | Sensitive to small changes in weights |
When it comes to solving one of the fundamental problems in computer science and network theory – finding the minimum spanning tree of a graph – two prominent algorithms step into the spotlight: Kruskal and Prim. These algorithms are like the dynamic duo of graph theory, working tirelessly to find the most efficient way to connect all the nodes in a graph without forming any cycles. But make no mistake; they have their unique approaches and nuances that set them apart. In this detailed exploration, we will compare Kruskal and Prim by one aspect: their key differences.
Differences Between Kruskal and Prim
The main differences between Kruskal and Prim algorithms lie in their approaches and applications. Kruskal, a weight-centric and edge-driven algorithm, excels in sparse graphs with varying edge weights, making it a go-to choice for network design and clustering tasks. In contrast, Prim, a vertex-centric approach, is best suited for dense graphs and scenarios where maintaining tree connectivity is paramount, making it ideal for applications in robotics, circuit design, and situations with highly connected data. The choice between Kruskal and Prim should be driven by your specific graph characteristics and the requirements of your problem.
1. Algorithm Approach
Kruskal:
Kruskal is an algorithm that takes a greedy approach to find the minimum spanning tree. It starts with an empty set of edges and iteratively adds edges to the tree in ascending order of their weights, while ensuring that adding each edge doesn’t form a cycle. This approach can be likened to growing the minimum spanning tree by picking the smallest available edge at each step, making it a great choice for sparse graphs.
Kruskal’s algorithm makes use of the disjoint-set data structure (also known as a union-find data structure) to efficiently detect cycles in the graph and determine whether adding an edge would create a cycle.
Prim:
In contrast, Prim’s algorithm also follows a greedy approach but with a different strategy. It starts with an arbitrary node as the initial tree and then repeatedly adds the edge with the smallest weight that connects a vertex in the tree to a vertex outside the tree. This process continues until all vertices are included in the minimum spanning tree. Prim’s approach is more like growing the tree from a single seed and gradually expanding it.
2. Edge Selection Criteria
Kruskal:
Kruskal’s algorithm selects edges solely based on their weight, without considering the relationship or connectivity of the nodes they connect. It sorts all edges by weight and adds them to the tree as long as they do not create a cycle.
This weight-centric approach makes Kruskal’s algorithm well-suited for graphs with edges of varying lengths and where the distribution of edge weights is relatively uniform.
Prim:
Prim’s algorithm, on the other hand, chooses edges based on their direct connection to the current minimum spanning tree. It always selects the edge with the minimum weight that connects a vertex in the tree to a vertex outside the tree. This ensures that the tree remains connected throughout the process.
Prim’s strategy is particularly efficient when dealing with graphs where the minimum spanning tree is expected to be highly connected and compact.
3. Complexity Analysis
Let’s dive into the computational complexities of Kruskal and Prim, which can greatly influence the choice between the two algorithms depending on the problem at hand.
Aspect | Kruskal | Prim |
---|---|---|
Time Complexity | O(E log E) | O(V^2) or O(E log V) |
Space Complexity | O(V + E) | O(V) |
Kruskal:
- Time Complexity: Kruskal’s algorithm has a time complexity of O(E log E), where E is the number of edges in the graph. This complexity arises from sorting all the edges by weight.
- Space Complexity: The algorithm requires additional space for storing the edges and disjoint-set data structures, resulting in a space complexity of O(V + E), where V is the number of vertices and E is the number of edges.
Prim:
- Time Complexity: Prim’s algorithm typically has a time complexity of O(V^2) when using an adjacency matrix to represent the graph. However, with the use of efficient data structures like binary heaps or Fibonacci heaps, this can be improved to O(E log V), where E is the number of edges and V is the number of vertices.
- Space Complexity: Prim’s algorithm has a space complexity of O(V) to store the vertices in the minimum spanning tree.
4. Suitable Graph Types
Both Kruskal and Prim are versatile algorithms that can be applied to a wide range of graph types, but they excel in different scenarios.
Kruskal:
Kruskal’s algorithm is particularly well-suited for graphs with the following characteristics:
- Sparse graphs where E (number of edges) is much less than V (number of vertices).
- When the graph has a wide range of edge weights, making it challenging to predict which edges will be included in the minimum spanning tree.
- Situations where the graph is already connected or nearly connected.
Prim:
Prim’s algorithm shines in graphs that exhibit the following characteristics:
- Dense graphs where E is close to V^2, as it can take advantage of adjacency matrices.
- When the graph is expected to have a compact minimum spanning tree with well-connected vertices.
- Situations where it’s crucial to maintain the connectivity of the tree throughout the process.
5. Parallelization Potential
Parallelization is a key consideration in modern computing, as it allows algorithms to leverage multiple processors or cores for faster execution. Let’s see how Kruskal and Prim fare in terms of parallelization potential.
Kruskal:
Kruskal’s algorithm has a natural tendency to be parallelized, especially during the sorting phase of edge weights. Sorting is a task that can be efficiently divided among multiple processing units. Therefore, Kruskal can benefit from parallelization, making it suitable for high-performance computing environments.
Prim:
Prim’s algorithm, while still amenable to parallelization to some extent, doesn’t have as clear-cut opportunities as Kruskal. The selection of edges in Prim depends on the current state of the minimum spanning tree, which makes parallelization more challenging. Nevertheless, there are parallel variants of Prim’s algorithm that can be employed.
6. Handling Negative Edge Weights
The ability to handle negative edge weights can be a crucial factor when choosing between Kruskal and Prim, depending on the nature of the problem.
Kruskal:
Kruskal’s algorithm can handle graphs with negative edge weights without any modifications. It will simply select the edges with the smallest absolute values first, effectively considering the magnitudes of the weights.
Prim:
Prim’s algorithm, as originally formulated, assumes that all edge weights are non-negative. Attempting to use it on graphs with negative edge weights could result in unexpected behavior or incorrect results. However, there are variations of Prim’s algorithm, such as the “Modified Prim’s Algorithm,” that can handle negative edge weights by introducing additional checks.
7. Applications
Both Kruskal and Prim algorithms find applications in various domains. Let’s explore some common and specific use cases for each algorithm.
Kruskal:
- Network Design: Kruskal is often used in designing communication networks, such as laying down fiber-optic cables or connecting cities with minimal cost.
- Clustering: It can be applied in data clustering, where the goal is to group data points based on similarity while minimizing the overall dissimilarity within each cluster.
- Molecular Chemistry: Kruskal’s algorithm can be used in molecular chemistry to determine the minimum energy required to form molecular structures.
- Image Segmentation: In image processing, Kruskal can assist in segmenting images by finding the most significant edges to create regions.
Prim:
- Computer Networking: Prim’s algorithm is frequently used in routing algorithms and network topology design, ensuring efficient data transmission.
- Robotics: It plays a crucial role in robotics for tasks like path planning and ensuring that robots reach their destinations with minimum traversal cost.
- Circuit Design: Prim’s algorithm can be applied in electronic circuit design to connect components efficiently while minimizing the overall cost.
- Geographic Information Systems (GIS): In GIS, Prim’s algorithm helps in creating efficient transportation networks or utility grids.
8. Handling Disconnected Components
Graphs aren’t always guaranteed to be fully connected, and this brings up an important consideration for Kruskal and Prim.
Kruskal:
Kruskal’s algorithm can handle graphs with disconnected components. It will find the minimum spanning forest, which consists of minimum spanning trees for each connected component in the graph. This ability can be advantageous in scenarios where disconnected components need to be separately optimized.
Prim:
Prim’s algorithm assumes that the input graph is connected. If applied to a graph with disconnected components, it will only find the minimum spanning tree for one of the components, starting from the initial seed node. To find the minimum spanning trees for all components, you would need to run Prim’s algorithm separately on each component.
9. Implementation Complexity
The ease of implementing an algorithm can greatly impact its practical use. Let’s compare the implementation complexity of Kruskal and Prim.
Kruskal:
Kruskal’s algorithm is relatively straightforward to implement. The core steps involve sorting edges by weight and iteratively adding them to the minimum spanning tree while ensuring that no cycles are formed. The use of a disjoint-set data structure simplifies cycle detection.
Prim:
Prim’s algorithm can be a bit more involved to implement due to the need to manage the connectivity of the tree. You must keep track of the vertices in the tree, as well as the vertices outside the tree. The selection of edges based on their connection to the tree requires careful bookkeeping.
10. Robustness to Edge Weight Perturbations
Real-world data can be noisy, and sometimes edge weights may not be perfectly accurate. It’s essential to consider how Kruskal and Prim respond to slight perturbations in edge weights.
Kruskal:
Kruskal’s algorithm is robust to small changes in edge weights. Since it selects edges purely based on their weights, minor perturbations are unlikely to significantly affect the final minimum spanning tree. This robustness can be advantageous in scenarios with noisy or imprecise data.
Prim:
Prim’s algorithm, due to its dependency on the current state of the tree, can be more sensitive to small changes in edge weights. A slight perturbation could lead to a different edge being chosen, potentially resulting in a different minimum spanning tree.
Kruskal or Prim : Which One is Right Choose for You?
Deciding whether to use Kruskal or Prim for your specific problem depends on various factors and the nature of your graph. To help you make an informed choice, let’s delve deeper into the considerations that can guide your decision-making process:
Choose Kruskal If:
1. Edge-Centric Approach:
- Sparse Graphs: Kruskal’s algorithm shines when dealing with sparse graphs, where the number of edges is much less than the number of vertices (E << V). It efficiently handles such scenarios.
2. Weight Flexibility:
- Varying Edge Weights: If your graph has edges with a wide range of weights, Kruskal is well-suited. It selects edges solely based on weight, making it adaptable to diverse weight distributions.
3. Parallelization:
- Parallel Computing: If you have access to parallel computing resources and want to leverage them effectively, Kruskal’s sorting phase can be efficiently parallelized, leading to faster execution.
4. Handling Negative Weights:
- Negative Edge Weights: If your problem involves graphs with negative edge weights, Kruskal can handle them without any modifications. It will consider the absolute values of the weights.
5. Implementation Ease:
- Simplicity: Kruskal’s algorithm is relatively straightforward to implement, making it an excellent choice when you need a quick and practical solution.
Choose Prim If:
1. Vertex-Centric Approach:
- Dense Graphs: Prim’s algorithm excels in dense graphs, where the number of edges is close to the square of the number of vertices (E ≈ V^2). It efficiently utilizes adjacency matrices in such cases.
2. Connectivity Matters:
- Connectedness: If maintaining the connectivity of the minimum spanning tree throughout the process is crucial for your application, Prim ensures a highly connected tree.
3. Limited Parallelization:
- Parallelization Challenges: While Prim can be parallelized to some extent, it is less straightforward than Kruskal. If parallelization is not a primary concern, this should not deter you from using Prim.
4. Handling Disconnected Components:
- Disconnected Graphs: If your graph may contain disconnected components, Prim can still find the minimum spanning tree for one of the components, making it a suitable choice when dealing with such graphs.
5. Specific Applications:
- Robustness to Weight Changes: If your data is noisy, and small perturbations in edge weights need to be handled carefully, Kruskal’s weight-centric approach may be less sensitive to these changes compared to Prim.
Making the Decision:
Ultimately, the choice between Kruskal and Prim depends on the unique characteristics of your problem and graph:
- Graph Density: Consider whether your graph is sparse or dense, as this can strongly influence the algorithm choice.
- Edge Weights: Examine the distribution of edge weights in your graph. Kruskal is more adaptable to varying weights, while Prim maintains high connectivity.
- Parallelization: If you have access to parallel computing resources and need a scalable solution, Kruskal may be more suitable.
- Connectivity: Assess the importance of maintaining connectivity throughout the process. Prim guarantees a highly connected tree.
- Handling Disconnected Components: If your graph may have disconnected components, Prim can handle this situation.
- Robustness: Evaluate how your algorithm choice responds to small changes in edge weights if your data is noisy.
In many cases, it’s beneficial to experiment with both algorithms on your specific data and problem to determine which one performs better. Remember that there is no one-size-fits-all answer, and the “right” choice ultimately depends on the intricacies of your particular scenario.
FAQs
Kruskal and Prim algorithms are used to find the Minimum Spanning Tree (MST) of a weighted graph. The MST is a subset of the graph’s edges that connects all vertices with the minimum possible total edge weight.
Kruskal’s algorithm works by iteratively adding edges to the MST in ascending order of their weights while ensuring that adding each edge does not create a cycle. It starts with an empty set of edges and grows the MST by selecting edges with the smallest weights.
Prim’s algorithm starts with an arbitrary node and repeatedly adds the edge with the smallest weight that connects a vertex in the MST to a vertex outside the MST. It continues until all vertices are included in the MST, gradually growing the tree from a single seed.
Kruskal’s algorithm is a good choice for sparse graphs with varying edge weights. It’s suitable for scenarios where you want to minimize the total edge weight while connecting all vertices. Common applications include network design, clustering, and image segmentation.
Prim’s algorithm is preferred for dense graphs or situations where maintaining high connectivity in the MST is essential. It works well with graphs where E (number of edges) is close to V^2 (number of vertices squared). Applications include robotics, circuit design, and geographical information systems (GIS).
Kruskal can handle negative edge weights naturally, considering the absolute values of weights. However, Prim, in its standard form, assumes non-negative edge weights. There are modified versions of Prim that can handle negative weights with additional checks.
The choice depends on factors like graph density, edge weight distribution, the need for connectivity, and the availability of parallel computing resources. Kruskal is suitable for sparse graphs and diverse weight distributions, while Prim excels in dense graphs and connectivity-sensitive applications.
Kruskal’s algorithm can be efficiently parallelized during the sorting phase, making it suitable for parallel computing environments. Prim’s parallelization is less straightforward but possible, especially when using efficient data structures.
Kruskal can find the Minimum Spanning Forest, which includes MSTs for each connected component in a disconnected graph. Prim, in its standard form, assumes a connected graph and will find the MST for one connected component.
Kruskal is generally more robust to small changes in edge weights because it selects edges based solely on weight values. Prim, due to its vertex-centric approach, can be more sensitive to slight changes in weights as they affect the tree’s structure.
Read More :
Contents