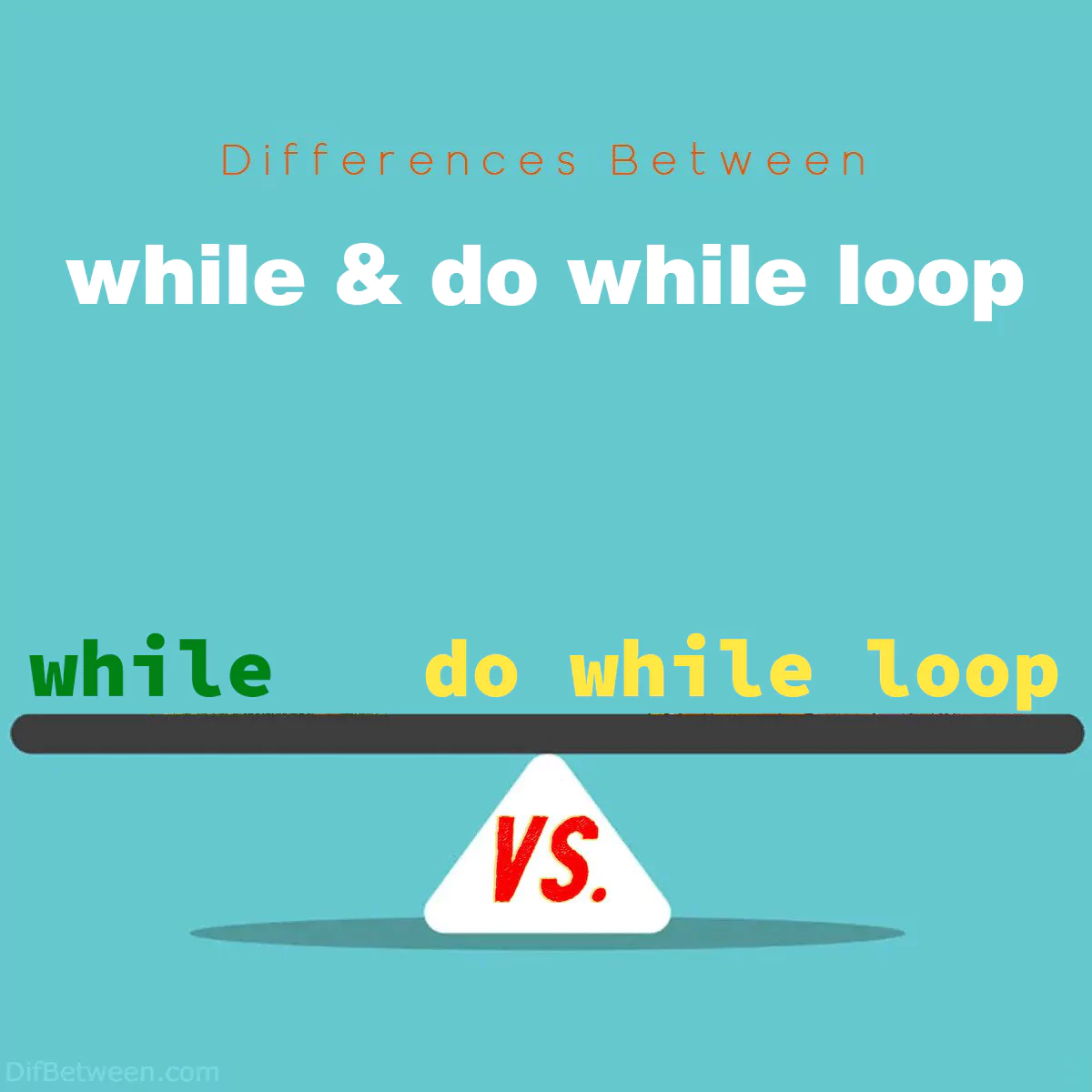
Aspect | while Loop | do-while Loop |
---|---|---|
Syntax | Starts with the condition. | Starts with the code block and ends with do followed by the condition. |
Initial Condition Check | Condition is checked before code execution. | Condition is checked after code execution. |
Use Cases | – Iterating over collections. | – Menu-driven programs. |
– Handling user input. | – Validation of user input. | |
– Countdowns and timers. | – Game loops. | |
Flow Control | – Pre-test loop. | – Post-test loop. |
– Condition checked before entering the loop. | – Code block executed at least once. | |
Conditional Differences | – Code may not run if the condition is false initially. | – Code block always runs at least once. |
Loop Termination | Occurs when the condition becomes false. | Also occurs when the condition becomes false. |
Loop may not run if the condition is false initially. | Code block always runs at least once. | |
Choosing Between | Use when the initial execution of the loop is not critical and the loop should run based on a condition. | Use when you need to ensure the code block runs at least once, regardless of the initial condition. |
In the world of programming, loops are like the unsung heroes, tirelessly repeating tasks until a certain condition is met. Among the many loop types, two stalwarts stand out: the trusty while
loop and its cousin, the do-while
loop. While they share similarities, they also have distinct differences. In this comprehensive guide, we’ll delve into the depths of these loops, exploring their unique aspects, similarities, and use cases. So, whether you’re a novice coder or a seasoned programmer, buckle up as we take a journey through the intriguing realm of while
and do-while
loops!
Differences Between while and do while loop
The primary distinction between a while
and a do-while
loop lies in when they evaluate their conditions. In a while
loop, the condition is checked before the code block execution, potentially resulting in zero iterations if the condition is initially false. Conversely, in a do-while
loop, the code block runs at least once before checking the condition, ensuring that it always executes initially, regardless of the condition’s initial state. This crucial difference in execution order dictates the choice between these two looping structures, depending on whether you need conditional execution from the start or require at least one iteration.
Structure and Syntax
while
Loop Syntax:
while (condition): # Code to be executed while the condition is true
do-while
Loop Syntax:
do { # Code to be executed at least once } while (condition);
The first difference between these two loops is their structure and syntax. The while
loop starts with the condition, which is checked before executing any code within the loop. If the condition is initially false, the loop may not run at all.
On the other hand, the do-while
loop begins with the code block enclosed in curly braces. This code block is guaranteed to execute at least once, regardless of whether the condition is true or false initially. After the code block is executed, the condition is checked, and if it evaluates to true, the loop continues to run.
Initial Condition Check
The fundamental distinction between these two loops lies in when they check the condition. This difference can be summarized as follows:
while
Loop: Condition is checked before the code block execution.do-while
Loop: Condition is checked after the code block execution.
Consider this analogy: Imagine you’re planning a picnic. In the case of a while
loop, you first check the weather forecast to decide whether to go out. If it’s raining (the condition is false), you stay home, and the picnic never happens. With a do-while
loop, you decide to go out for a picnic first and then check the weather (the condition) afterward. This means you’ll have your picnic even if it starts raining later, as you’ve already made the initial commitment.
Use Cases
while
Loop Use Cases
The while
loop is versatile and widely used. It’s primarily employed when you want to repeat a block of code as long as a certain condition is met. Some common use cases for while
loops include:
Iterating Over Collections:
You can use a while
loop to traverse elements in an array, list, or any collection type based on a condition. For example, you might iterate through a list of students and stop when you find a student with a specific GPA.
Handling User Input
In interactive applications, while
loops are handy for continually prompting users for input until they provide valid data. This ensures that your program doesn’t progress until it receives the desired input.
Countdowns and Timers
Creating countdowns or timers is another use case for while
loops. You can decrement a counter in each iteration and execute specific actions when it reaches zero.
do-while
Loop Use Cases
The do-while
loop may not be as commonly used as its counterpart, but it has its niche. It’s best suited for scenarios where you need to execute a block of code at least once, regardless of the condition’s initial state. Some common use cases for do-while
loops include:
Menu-Driven Programs
In menu-driven console applications, you often want to display the menu at least once to allow the user to make a choice. A do-while
loop ensures that the menu is shown before checking the user’s input.
Validation
When validating user input, a do-while
loop can be valuable. It guarantees that the validation logic runs at least once, preventing the program from moving forward with invalid input.
Game Loops
In game development, you might have a game loop where you update the game state and render graphics repeatedly. A do-while
loop can ensure that the game loop runs at least once to initialize the game state.
Flow Control
The order in which the condition is checked and the code is executed can significantly impact the flow of your program. Let’s dive deeper into this aspect.
while
Loop Flow Control
In a while
loop, the condition is checked before the code block execution. If the condition is initially false, the code block is never executed. Here’s a step-by-step breakdown of the flow:
- Check the condition.
- If the condition is true, execute the code block.
- After executing the code block, return to step 1.
- If the condition is false, exit the loop.
In essence, the while
loop is a “pre-test” loop, as it checks the condition before entering the loop.
do-while
Loop Flow Control
The do-while
loop, in contrast, is a “post-test” loop. It executes the code block first and then checks the condition. Here’s how the flow unfolds:
- Execute the code block.
- Check the condition.
- If the condition is true, return to step 1.
- If the condition is false, exit the loop.
This flow guarantees that the code block runs at least once, making it a useful choice for situations where initial execution is crucial.
Conditional Differences
The way conditions are evaluated in while
and do-while
loops can lead to variations in behavior. Let’s explore these conditional differences.
while
Loop Condition
In a while
loop, the condition is checked before the code block execution. If the condition is initially false, the loop is never entered, and the code inside it doesn’t run at all.
Here’s a table summarizing the behavior:
Initial Condition | Loop Execution |
---|---|
Condition is true | Code runs |
Condition is false | Code doesn’t run |
do-while
Loop Condition
In a do-while
loop, the condition is checked after the code block execution. This means that the code block always runs at least once, regardless of the initial condition.
Here’s a table summarizing the behavior:
Initial Condition | Loop Execution |
---|---|
Condition is true | Code runs |
Condition is false | Code runs once, then exits |
Loop Termination
Both while
and do-while
loops have mechanisms for termination, but the conditions for termination are evaluated at different points in the loop’s execution.
while
Loop Termination
In a while
loop, termination occurs when the condition becomes false. If the condition is initially false, the loop may not run at all.
do-while
Loop Termination
In a do-while
loop, termination also occurs when the condition becomes false. However, since the condition is checked after the code block execution, the code block always runs at least once.
while or do while loop : Which One is Right Choose for You?
Choosing between a while
loop and a do-while
loop depends on your specific programming requirements and the desired flow of execution in your code. Let’s explore when to use each type of loop:
When to Use a while
Loop:
Condition-Dependent Execution:
- Use a
while
loop when you want to repeat a block of code based on a condition. - This loop is suitable when the execution of the loop depends on the initial state of the condition.
- It’s a “pre-test” loop because the condition is checked before entering the loop. If the condition is initially false, the loop may not run at all.
Iterating Over Collections:
while
loops are often used to traverse elements in arrays, lists, or other collection types based on a condition.- For example, you might iterate through a list of items and stop when you find a specific item that meets a certain criteria.
Handling User Input:
- In interactive applications,
while
loops are useful for continually prompting users for input until valid data is provided. - This ensures that your program doesn’t progress until it receives the expected input.
Countdowns and Timers:
- Creating countdowns or timers is another use case for
while
loops. - You can decrement a counter in each iteration and execute specific actions when it reaches zero.
When to Use a do-while
Loop:
Initial Execution Guarantee:
- Use a
do-while
loop when you need to ensure that a block of code runs at least once, regardless of the initial state of the condition. - This loop is valuable when you want to execute a specific action before checking a condition.
- It’s a “post-test” loop because the code block always runs at least once before the condition is checked.
Menu-Driven Programs:
- In menu-driven console applications, you often want to display the menu at least once to allow the user to make a choice.
- A
do-while
loop ensures that the menu is shown before checking the user’s input.
Validation:
- When validating user input, a
do-while
loop can be beneficial. - It guarantees that the validation logic runs at least once, preventing the program from moving forward with invalid input.
Game Loops:
- In game development, you might have a game loop where you update the game state and render graphics repeatedly.
- A
do-while
loop can ensure that the game loop runs at least once to initialize the game state.
In summary, the choice between a while
loop and a do-while
loop should be based on the specific requirements of your program and the desired flow of execution. If your code can operate without the initial execution of the loop, and the condition determines whether the loop should run, opt for a while
loop. On the other hand, if you need to guarantee initial execution of a block of code, even before checking the condition, a do-while
loop is the better choice. Understanding these distinctions will help you write more efficient and effective code in your programming endeavors.
FAQs
while
loop in programming? A while
loop is a control structure in programming that repeatedly executes a block of code as long as a specified condition remains true.
do-while
loop? A do-while
loop is another type of control structure that also repeats a block of code based on a condition. However, in a do-while
loop, the code block is guaranteed to execute at least once before the condition is checked.
while
and do-while
loops? The key distinction lies in when the condition is checked. In a while
loop, the condition is evaluated before the code block, potentially resulting in zero iterations. In a do-while
loop, the code block executes at least once before checking the condition.
while
loop? Use a while
loop when your code can operate without the initial execution of the loop, and you want the condition to determine whether the loop should run or not.
do-while
loop more suitable? Choose a do-while
loop when you need to ensure that a block of code runs at least once, regardless of the initial condition state. This is useful for scenarios like input validation and menu-driven programs.
while
loop? Certainly! A common example is iterating through an array or list until a specific condition is met, such as finding a target element.
do-while
loop? A typical use case is a menu-driven program where you want to display the menu at least once, even if the user’s first choice is to exit the program.
while
and do-while
loops available in all programming languages? Yes, while
and do-while
loops are fundamental constructs and are available in most programming languages, including C++, Java, Python, and many others.
Generally, the performance difference between while
and do-while
loops is negligible. The choice should be based on the logic and flow of your program rather than performance considerations.
while
or do-while
loops within each other? Yes, you can nest while
or do-while
loops inside other loops, as long as you maintain proper control flow and exit conditions for each loop.
Read More :
Contents