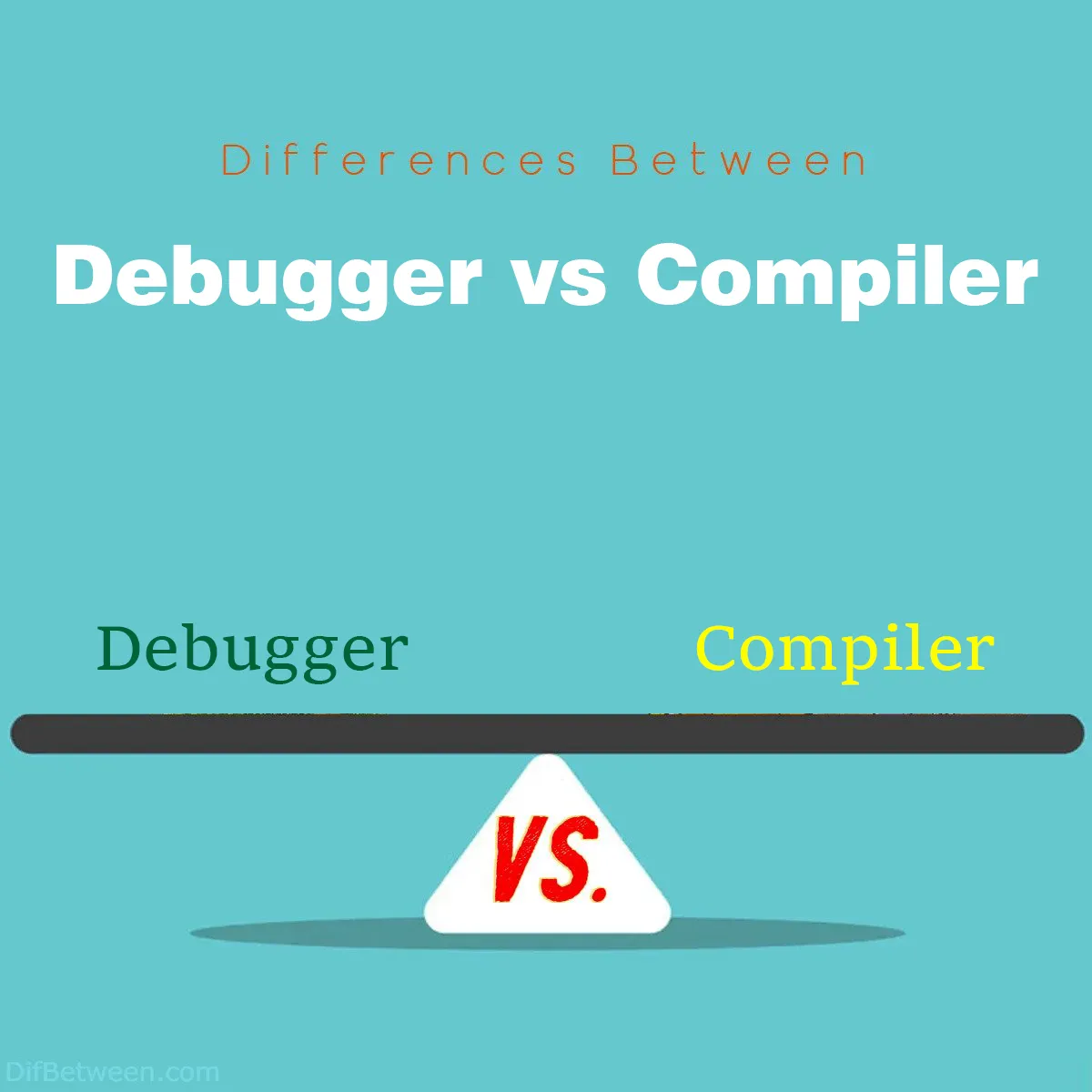
Aspect | Debugger | Compiler |
---|---|---|
Purpose | Identifying and fixing bugs and issues in code. | Translating source code into machine code or bytecode. |
Timing of Use | During development and testing phases. | After development, for deployment or distribution. |
Output | Information about code behavior (variables, stack, errors). | Executables, bytecode, libraries, or error messages. |
Interaction | Highly interactive, allowing control over code execution. | Operates in batch mode with limited user interaction. |
Granularity | Fine-grained control over code execution (breakpoints, step-through). | Processes entire source code as a single unit. |
Primary Goal | To locate and resolve issues in code. | To generate machine-readable output. |
Features | – Breakpoints\n- Variable Inspection\n- Call Stack Examination\n- Conditional Breakpoints\n- Step-Through Execution\n- Watchlists | – Optimization Levels\n- Cross-Compilation\n- Static Analysis\n- Linking\n- Standard Compliance |
Advantages | – Efficient Bug Hunting\n- Precise Code Inspection\n- Interactive Learning\n- Better Code Quality | – Performance Optimization\n- Platform Independence\n- Code Protection\n- Efficient Distribution |
Popular Tools | – GDB (GNU Debugger)\n- Visual Studio Debugger\n- Xcode Debugger\n- PyCharm Debugger\n- Chrome DevTools | – GCC (GNU Compiler Collection)\n- Clang\n- Java Compiler (javac)\n- Microsoft C# Compiler (csc)\n- Python Compiler (CPython) |
When it comes to software development, two essential tools play distinct but equally crucial roles: the debugger and the compiler. These tools are pivotal in the software development process, helping programmers catch errors and convert human-readable code into machine-executable instructions. In this article, we’ll explore the key differences between a debugger and a compiler, shedding light on how they function, their purposes, and when and why you’d use one over the other.
Differences Between Debugger and Compiler
Debugger vs Compiler: The main differences lie in their roles and timing within the software development process. A debugger is a tool used during the development and testing phases, serving as a detective to identify and rectify bugs, logical errors, and issues in the code. It provides a high level of interactivity, enabling developers to pause code execution, inspect variables, and step through the code. In contrast, a compiler steps into action after development, translating human-readable source code into machine-executable instructions or bytecode, promoting platform independence and performance optimization. While debuggers help find and fix problems in real-time, compilers ensure code is ready for efficient deployment across various platforms.
Purpose and Function
Debugger
A debugger is like a detective for your code, helping you uncover and resolve issues within your program. Its primary purpose is to assist developers in identifying and fixing bugs, glitches, and logical errors in their code. Think of it as a magnifying glass for your codebase, allowing you to scrutinize every line and variable to understand what’s going wrong.
How it Works
Debuggers work by allowing developers to pause the execution of a program at specific breakpoints or lines of code. At these breakpoints, you can inspect the current state of your program, view the values of variables, and step through the code one line at a time. This step-by-step analysis is immensely helpful in tracking down the root cause of issues.
In addition to breakpoints, debuggers often provide features like watchlists, call stack inspection, and the ability to set conditional breakpoints. These features give developers granular control over the debugging process, making it easier to pinpoint and fix problems.
Compiler
On the other hand, a compiler has a completely different role in the software development process. Its primary purpose is to translate the human-readable source code written by developers into machine code or bytecode that the computer’s processor can understand and execute. In essence, a compiler bridges the gap between high-level programming languages like Java, C++, or Python and the binary code that a computer can run.
How it Works
Compilers follow a multi-step process to convert source code into machine code:
- Lexical Analysis: The compiler breaks down the source code into tokens, identifying keywords, variables, and symbols.
- Syntax Analysis: It checks the structure of the code to ensure it conforms to the language’s grammar and rules. If any syntax errors are found, the compiler reports them.
- Semantic Analysis: The compiler verifies that the code makes sense semantically, checking for type mismatches, undefined variables, or other logical errors.
- Intermediate Code Generation: Some compilers generate an intermediate representation of the code before producing machine code. This step can optimize the code further.
- Code Optimization (Optional): In some cases, compilers perform optimization techniques to make the resulting machine code more efficient.
- Code Generation: Finally, the compiler translates the code into machine code or bytecode that can be executed by the computer.
In summary, while a debugger helps you find and fix issues in your code, a compiler translates your code into a format the computer can execute.
Timing of Use
Debugger
Debuggers are primarily used during the development and testing phases of software development. When you’re writing code and encountering issues or unexpected behavior, you can fire up a debugger to investigate and resolve the problems.
Typical Use Cases
- Bug Hunting: Debuggers are invaluable when you encounter runtime errors, crashes, or unexpected behavior in your program. They allow you to pinpoint the exact location and cause of the problem.
- Stepping Through Code: When you want to understand how your code executes step by step, a debugger is your best friend. It helps you visualize the flow of your program.
- Unit Testing: Debuggers are often used in conjunction with unit testing to verify that individual components or functions of your code work as expected.
Compiler
Compilers are used primarily when you’re ready to deploy or distribute your software. They come into play after you’ve written, tested, and debugged your code. Compilers transform your high-level code into machine code that can be run independently of the development environment.
Typical Use Cases
- Code Deployment: When you’re ready to share your software with others or run it on different machines, you’ll use a compiler to create an executable or bytecode version of your program.
- Platform Independence: Compilers allow you to write code in a high-level language and then compile it for various platforms. This is particularly important for cross-platform development.
- Optimization: Compilers can optimize your code for performance, potentially making it run faster and more efficiently than the original source code.
Output
Debugger
The output of a debugger is information and insights about your code’s runtime behavior. Debuggers provide a user interface that displays various pieces of information, including:
- Variable Values: You can inspect the current values of variables, which is crucial for understanding why your code behaves a certain way.
- Call Stack: Debuggers show you the call stack, allowing you to trace how functions or methods were called and in what order.
- Breakpoint Hit Points: When a breakpoint is hit, the debugger notifies you, indicating which line of code caused the break.
- Error Messages: If an error occurs during debugging, you’ll receive detailed error messages that can help you diagnose the issue.
- Console Output: Debuggers often provide a console or output window where you can print custom messages for debugging purposes.
Compiler
The output of a compiler is typically an executable file, a library, or bytecode, depending on the programming language and the compiler used. Let’s take a closer look at the types of outputs you can expect:
- Executable Files: In languages like C or C++, the compiler generates standalone executable files that can be run directly on a computer or server.
- Bytecode: In languages like Java, the compiler produces bytecode, which is an intermediate representation that can be executed by the Java Virtual Machine (JVM). This bytecode is platform-independent.
- Dynamic Link Libraries (DLLs): Compilers can also produce dynamic link libraries in languages like C#. These libraries are loaded at runtime and contain reusable code components.
- Intermediate Code: Some compilers generate an intermediate representation of the code, which can be further processed or optimized by another tool.
- Error Messages: Like debuggers, compilers provide error messages if they encounter issues during the compilation process. These messages help you identify and fix problems in your source code before generating the final output.
Interaction with Code
Debugger
Debuggers are highly interactive tools that allow developers to control the execution of their code. Here are some key ways in which developers interact with debuggers:
- Setting Breakpoints: Developers can set breakpoints at specific lines of code where they want the program to pause. This is particularly useful for isolating and investigating issues.
- Stepping Through Code: Debuggers provide options to step through the code, whether it’s line-by-line or by jumping to the next breakpoint or function call.
- Variable Inspection: Developers can inspect the values of variables in real-time, helping them understand how data changes as the code runs.
- Conditional Breakpoints: Debuggers allow developers to set conditions for breakpoints, so they only trigger when specific criteria are met. For example, a breakpoint might only activate if a variable exceeds a certain value.
- Watchlists: Developers can create watchlists to monitor the values of specific variables continuously while debugging.
Compiler
In contrast to debuggers, compilers are not interactive tools in the same way. They operate in a batch mode, processing the entire source code and generating output. Developers typically interact with compilers through command-line arguments or configuration files.
Here’s how developers interact with compilers:
- Compilation Command: Developers initiate the compilation process by executing a command that specifies the source code file(s), target platform, and any compiler flags or options.
- Configuration Files: In some cases, developers use configuration files to specify compilation settings, such as optimization levels or inclusion of external libraries.
- Error Handling: If the compiler encounters errors in the source code, it provides error messages and potentially stops the compilation process. Developers must address these errors before successfully compiling the code.
- Compilation Output: Once the compilation process is complete, the compiler generates the output (executable, bytecode, or library), which developers can then run or distribute.
Debugger or Compiler : Which One is Right TO Choose?
Choosing between a debugger and a compiler depends on the stage of your software development process and your specific goals. These two tools serve distinct purposes and are often used at different points in the development lifecycle. Here’s a breakdown to help you decide which one to choose:
Debugger: When to Choose
- During Development and Testing: Debuggers are primarily used during the development and testing phases when you’re actively writing and debugging your code.
- Identifying and Fixing Bugs: Use a debugger when you need to locate and resolve issues in your code, such as runtime errors, crashes, or unexpected behavior.
- Understanding Code Flow: Debuggers are essential when you want to understand how your code flows and why certain variables have specific values.
- Interactive Exploration: Debuggers allow you to interactively explore and experiment with your code, making them invaluable for learning and troubleshooting.
- Unit Testing: Debuggers are often used alongside unit testing frameworks to verify that individual code units (functions or methods) work as expected.
Compiler: When to Choose
- After Development for Deployment: Compilers come into play after the development and debugging phases when you’re ready to deploy or distribute your software.
- Platform Independence: Use a compiler when you want your code to run on multiple platforms without modification. Compilers can generate platform-independent bytecode or executables.
- Performance Optimization: Compilers are essential for optimizing your code for better performance, potentially making it run faster and more efficiently than the original source code.
- Code Protection: If you want to protect your source code from being easily reverse-engineered, use a compiler to create compiled binaries.
- Efficient Distribution: Compilers allow you to distribute your software without exposing the original source code, making them suitable for commercial applications.
In summary, debuggers are your go-to tool when actively writing and debugging code, while compilers are used to translate and optimize code for deployment. They are complementary tools in the software development process, and the choice between them depends on your current development phase and objectives.
FAQs
A debugger is a software tool used by developers to identify and resolve issues in their code during the development and testing phases. It allows developers to pause code execution, inspect variables, and step through code to understand its behavior and locate bugs.
A compiler is a software tool that translates human-readable source code into machine-executable instructions or bytecode. It bridges the gap between high-level programming languages and the binary code that a computer can run. Compilers are used to optimize code for performance and create platform-independent executables.
Debuggers are best used during the development and testing phases when you are actively writing and debugging code. They are essential for identifying and fixing bugs, understanding code flow, and ensuring the correctness of your software.
Compilers are used after the development phase, primarily for deploying or distributing software. They are employed to create efficient and platform-independent executables, optimize code for performance, and protect source code.
Yes, there are several popular debugger tools available, including GDB (GNU Debugger), Visual Studio Debugger, Xcode Debugger, PyCharm Debugger, and Chrome DevTools, among others. The choice of debugger depends on your programming language and development environment.
Popular compiler tools include GCC (GNU Compiler Collection), Clang, Java Compiler (javac), Microsoft C# Compiler (csc), and Python Compiler (CPython). The choice of compiler depends on the programming language you are using.
Yes, debuggers and compilers are complementary tools in software development. Developers often use debuggers during the coding and testing phases to find and fix issues, and then use compilers to generate optimized and distributable code once the development is complete.
Debuggers help ensure code correctness by identifying and resolving bugs, while compilers contribute to software quality by optimizing code for performance and facilitating efficient distribution.
Yes, compiled code is typically harder to reverse engineer compared to source code, providing a level of protection for your intellectual property.
Yes, many modern IDEs integrate both debugging and compilation features, making it convenient for developers to use both tools within a single development environment. Examples include Visual Studio, Xcode, and PyCharm, among others.
Read More :
Contents