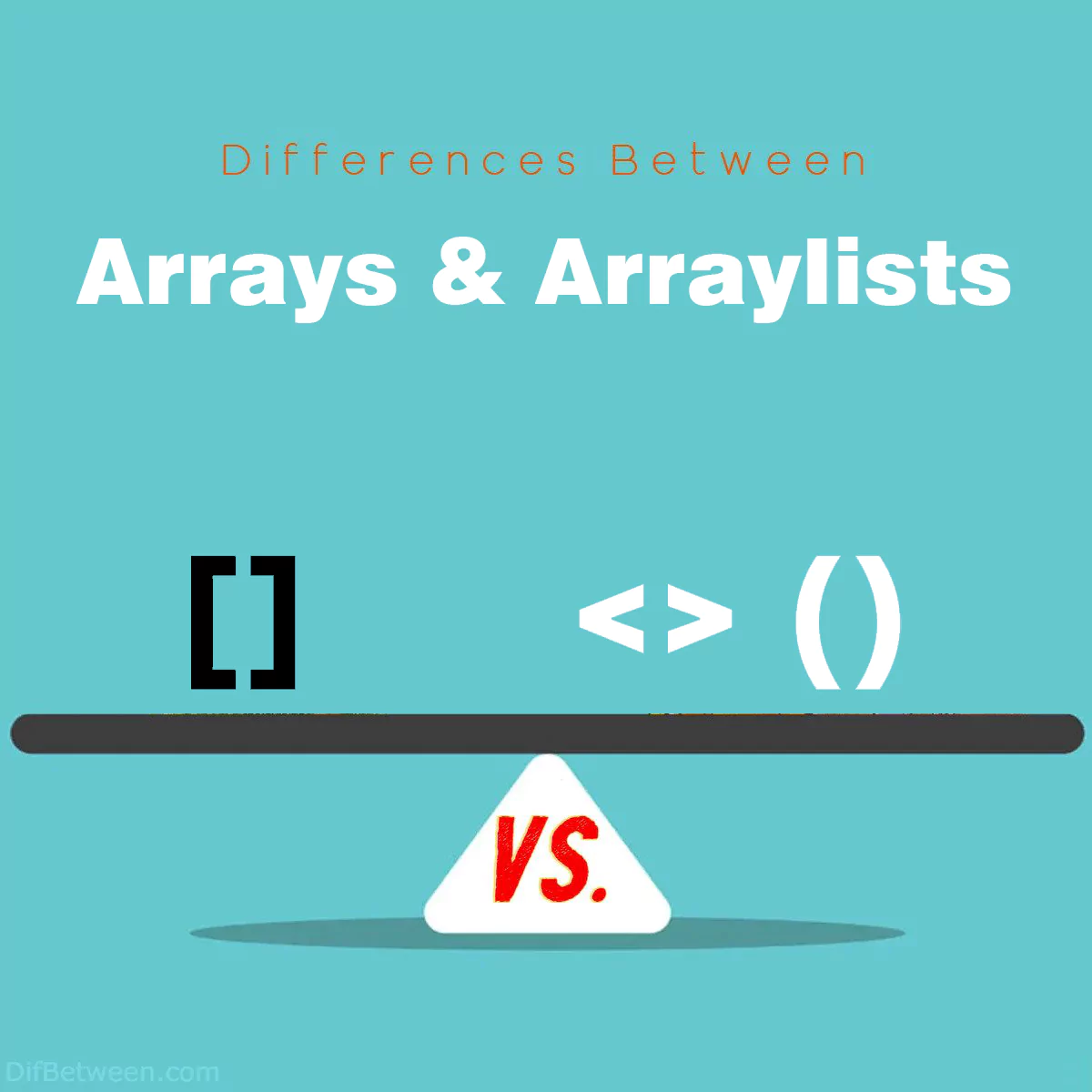
Size Flexibility | Fixed size; cannot be changed after creation. | Dynamic resizing; can grow or shrink as needed. |
Data Types | Homogeneous; elements must be of the same type. | Heterogeneous; can store elements of different types. |
Access Time | Fast and constant time for random access. | Slightly slower due to resizing overhead. |
Memory Usage | Memory allocated precisely for declared size. | Memory allocation based on current size and reserved space. |
Type Safety and Compile-Time Checks | Strong type safety enforces data type consistency. | Less compile-time type safety due to Object-based structure. |
Initialization | Requires specifying size and initializing elements. | Initialization without specifying size is possible. |
Adding Elements | Fixed size; must create a new larger array for additions. | Dynamically adjusts size as elements are added. |
Removing Elements | Not straightforward; may require shifting elements. | Elements can be easily removed, and the list shrinks accordingly. |
Performance Trade-offs | Excellent for fixed-size collections with known size. | Better suited for dynamic collections with varying sizes. |
Suitable Use Cases | When the size is known and fixed in advance. | When size is uncertain or varies during runtime. |
Compile-Time Errors | Type mismatch errors caught at compile time. | Type-related errors may surface at runtime if not handled correctly. |
In the world of programming, arrays and ArrayLists are two essential tools that developers frequently use to store and manage collections of data. While they share some similarities, they also have distinct differences that make each of them suitable for specific scenarios. In this guide, we will dive into the key differences between arrays and ArrayLists, helping you understand when to choose one over the other.
Differences Between Arrays and Arraylists
The main differences between Arrays and ArrayLists lie in their flexibility, data types, and performance. Arrays have a fixed size and enforce homogeneity, meaning they can only store elements of the same data type, providing fast and predictable access but requiring manual resizing and strong type safety. On the other hand, ArrayLists offer dynamic resizing and the ability to store heterogeneous data types, making them flexible for varying collection sizes. However, they may have slightly slower access times due to resizing overhead and offer less compile-time type safety. The choice between Arrays and ArrayLists depends on your specific project requirements, with Arrays excelling in fixed-size scenarios and ArrayLists in dynamic, flexible ones.
Overview of Arrays and ArrayLists
Before delving into their differences, let’s take a moment to understand what arrays and ArrayLists are.
Arrays
Arrays are a fundamental data structure in most programming languages. They provide a way to store a fixed-size collection of elements of the same data type. Elements in an array are accessed by their index, which is a numerical value representing their position in the array. Arrays offer efficient memory usage and fast access times, but their size is static, meaning it cannot change after initialization.
ArrayLists
ArrayLists, on the other hand, are a part of the Java Collections Framework and are not available in all programming languages. They belong to the class hierarchy of Java’s collection framework and offer dynamic resizing, allowing you to add or remove elements without specifying the size beforehand. ArrayLists provide greater flexibility compared to arrays but may come with some performance trade-offs.
Now, let’s explore the key differences between these two data structures in more detail.
Flexibility and Size
One of the most significant differences between arrays and ArrayLists is their flexibility regarding size.
Arrays
Arrays have a fixed size, which means that once you declare and initialize an array, you cannot change its size. If you need to store more elements than the array’s size allows, you must create a new, larger array and copy the elements from the old one to the new one. This process can be cumbersome and less efficient in terms of memory management.
Here’s a visual representation of how arrays handle size:
Index | 0 | 1 | 2 | 3 |
---|---|---|---|---|
Value | 10 | 20 | 30 | 40 |
If you want to add another element (e.g., 50) to this array, you would need to create a new, larger array, like this:
Index | 0 | 1 | 2 | 3 | 4 |
---|---|---|---|---|---|
Value | 10 | 20 | 30 | 40 | 50 |
ArrayLists
ArrayLists, on the other hand, provide dynamic resizing. You don’t need to specify their size during initialization, and they automatically grow or shrink as you add or remove elements. This flexibility makes ArrayLists more convenient when you’re uncertain about the number of elements you’ll be storing.
Here’s how an ArrayList handles size:
Index | 0 | 1 | 2 | 3 |
---|---|---|---|---|
Value | 10 | 20 | 30 | 40 |
Adding a new element (e.g., 50) to an ArrayList is straightforward, as it can automatically adjust its size:
Index | 0 | 1 | 2 | 3 | 4 |
---|---|---|---|---|---|
Value | 10 | 20 | 30 | 40 | 50 |
In summary, arrays have a fixed size, while ArrayLists can dynamically resize to accommodate varying numbers of elements.
Data Types and Homogeneity
Another significant difference between arrays and ArrayLists lies in the data types they can hold and their homogeneity.
Arrays
Arrays are homogeneous, meaning they can only store elements of the same data type. For example, if you declare an array of integers, you can only store integers in that array. This strict data type enforcement ensures consistency but can be limiting when you need to store different types of data within the same collection.
ArrayLists
ArrayLists, on the other hand, are more flexible in terms of data types. They can hold elements of different data types because they are based on Java’s Object class. This flexibility allows you to store heterogeneous data in an ArrayList, making it a versatile choice when you need to work with diverse data types.
Here’s a comparison:
Arrays (Homogeneous)
int[] intArray = new int[5]; intArray[0] = 10; intArray[1] = 20; intArray[2] = 30; // Attempting to add a non-integer element would result in a compilation error.
ArrayLists (Heterogeneous)
ArrayList<Object> mixedList = new ArrayList<>(); mixedList.add(10); mixedList.add("Hello"); mixedList.add(3.14);
In the ArrayList example, we can see that we are freely mixing integers, strings, and floating-point numbers in the same list, which is not possible with arrays.
In essence, arrays are strictly homogeneous, while ArrayLists allow for heterogeneous collections.
Performance Considerations
Performance is a crucial factor when choosing between arrays and ArrayLists, and their behavior differs in several aspects.
Arrays
Arrays generally offer better performance in terms of access time because elements are stored in contiguous memory locations. This allows for efficient random access using index values. Whether you want to retrieve the first or last element, or any element in between, the access time remains constant.
However, as mentioned earlier, arrays have a fixed size, and resizing requires creating a new array and copying elements. This operation can be expensive in terms of time and memory when the array needs to grow.
ArrayLists
ArrayLists provide dynamic resizing, making them more convenient for scenarios where the number of elements varies. However, this flexibility comes at the cost of slightly reduced performance compared to arrays. The dynamic resizing involves behind-the-scenes operations to allocate new memory and copy elements when the ArrayList reaches its capacity. This overhead can lead to slower performance when compared to arrays for some operations.
Here’s a basic performance comparison:
Arrays (Fast Access, Fixed Size)
- Fast random access (constant time).
- Fixed size requires manual resizing, which can be costly.
ArrayLists (Dynamic Size, Slightly Slower Access)
- Dynamic resizing to accommodate varying numbers of elements.
- Slightly slower access times due to resizing overhead.
In summary, if you require fast and predictable access times and know the size of your collection in advance, arrays may be the better choice. However, if you need flexibility in size and are willing to accept slightly slower access times, ArrayLists provide a more convenient option.
Memory Usage
Memory consumption is another critical aspect where arrays and ArrayLists differ.
Arrays
Arrays are memory-efficient because they have a fixed size determined at the time of creation. Since the size is known, memory allocation is straightforward, and there is no wasted memory. However, if the array’s size is set too large, you may end up with unused memory.
ArrayLists
ArrayLists, by their dynamic nature, can lead to more memory consumption. They allocate memory based on their current size, but they may also reserve extra space to accommodate future additions without frequent reallocations. This can result in some wasted memory if the ArrayList doesn’t reach its maximum capacity.
Here’s a comparison:
Arrays (Efficient Memory Usage)
- Memory is allocated precisely for the declared size.
- No wasted memory, but potential for underutilization if the size is too large.
ArrayLists (Dynamic Memory Allocation, Potential Wastage)
- Memory allocation depends on the current size and potential reserved space.
- Some memory may be wasted due to reserved space, but it allows for dynamic resizing.
In practice, if memory efficiency is a top priority, and you have a fixed-size collection, arrays are a more suitable choice. ArrayLists, on the other hand, provide flexibility at the cost of potentially higher memory usage.
Type Safety and Compile-Time Checks
Type safety and compile-time checks are essential considerations in programming, especially when dealing with large codebases.
Arrays
Arrays offer strong type safety and compile-time checks. The compiler enforces that you can only store elements of the declared data type in the array. This ensures that your code is less prone to runtime errors related to data type mismatches.
ArrayLists
ArrayLists, due to their flexibility with heterogeneous data types, offer less compile-time type safety. Since ArrayLists are based on the Object class, you can add elements of different data types without immediate compiler errors. However, this flexibility comes at the cost of potential runtime errors if you don’t handle type checking and casting correctly.
Here’s a comparison:
Arrays (Strong Type Safety)
int[] intArray = new int[5]; intArray[0] = 10; intArray[1] = "Hello"; // Compilation error: Incompatible types
ArrayLists (Less Compile-Time Type Safety)
ArrayList<Object> mixedList = new ArrayList<>(); mixedList.add(10); mixedList.add("Hello"); // Compiles successfully but may lead to runtime errors.
In summary, if type safety and catching errors at compile time are crucial for your project, arrays provide stronger guarantees. ArrayLists, while flexible, require careful handling to ensure type safety.
Arrays or Arraylists: Which One is Right Choose for You?
When it comes to managing collections of data in your code, you often have to make a decision: should you use arrays or ArrayLists? Both of these data structures have their strengths and weaknesses, and the choice between them depends on the specific requirements of your project. In this guide, we’ll help you make an informed decision by highlighting the scenarios where each of these options shines.
Arrays: The Fixed-Size Workhorses
Arrays are like the dependable workhorses of the programming world. They offer excellent performance for scenarios where you know the size of your collection in advance and don’t need to change it dynamically. Here are some situations where arrays are the right choice:
1. Fixed and Known Size
If you have a collection of elements with a fixed and known size, using an array is efficient. Arrays allocate memory precisely for the declared size, ensuring no memory is wasted. This makes them perfect for situations where the size is constant throughout the program’s execution.
2. Fast and Predictable Access
Arrays provide fast and predictable access times. Whether you need to retrieve the first element or access an element by its index, the access time remains constant. If your application relies on quick and efficient data retrieval, arrays are a solid choice.
3. Strong Type Safety
Arrays offer strong compile-time type safety. The compiler enforces that you can only store elements of the declared data type in the array, reducing the risk of runtime errors related to data type mismatches. This can be especially valuable in large codebases where catching errors at compile time is essential.
4. Memory Efficiency
For fixed-size collections, arrays are memory-efficient. They allocate memory precisely for the declared size, ensuring minimal memory usage. This makes them ideal for situations where memory optimization is a priority.
However, keep in mind that arrays are inflexible when it comes to resizing. If your collection’s size needs to change dynamically, you may face challenges with arrays.
ArrayLists: The Dynamic and Flexible Choice
ArrayLists, part of the Java Collections Framework, provide the flexibility that arrays lack. They are a dynamic choice, allowing you to add or remove elements without specifying the size beforehand. Here’s when ArrayLists are the right choice:
1. Dynamic Sizing
When your collection’s size varies during runtime, ArrayLists shine. They can grow or shrink as needed, automatically handling resizing operations. This flexibility makes ArrayLists well-suited for scenarios where the number of elements is uncertain or changes over time.
2. Heterogeneous Data
ArrayLists can store elements of different data types, making them versatile for handling heterogeneous data. If your collection needs to accommodate various data types, ArrayLists offer the flexibility you need.
3. Convenient Initialization
ArrayLists allow for initialization without specifying a size, which can simplify your code. You can start with an empty ArrayList and add elements as needed, avoiding the need to predict the initial size.
4. Easy Element Removal
If you frequently need to remove elements from your collection, ArrayLists provide a straightforward solution. Elements can be easily removed, and the list automatically shrinks accordingly, reducing memory wastage.
However, it’s important to note that ArrayLists may introduce slight performance trade-offs due to their dynamic resizing. If you prioritize fast and predictable access times or are working with a fixed-size collection, arrays might be the better option.
Making the Choice
In summary, the choice between arrays and ArrayLists boils down to your project’s specific needs:
- Use Arrays When:
- You have a fixed and known size.
- Fast and predictable access times are crucial.
- Strong compile-time type safety is required.
- Memory efficiency is a priority.
- Use ArrayLists When:
- Your collection’s size varies during runtime.
- You need to handle heterogeneous data types.
- Convenient initialization and easy element removal are essential.
- You are willing to accept slightly slower access times for flexibility.
Ultimately, there’s no one-size-fits-all answer. The right choice depends on the unique demands of your project. By considering factors like size flexibility, access speed, data type requirements, and memory usage, you can confidently choose between arrays and ArrayLists to achieve the best results in your programming endeavors.
FAQs
An array is a fixed-size data structure that stores a collection of elements of the same data type. Elements in an array are accessed by their index, which is a numerical value representing their position in the array.
An ArrayList is a dynamic data structure in Java that belongs to the Java Collections Framework. It can grow or shrink in size as elements are added or removed. Unlike arrays, ArrayLists can store elements of different data types.
Arrays are best suited for scenarios where you have a fixed and known collection size, and you need fast and predictable access times. They are also ideal when strong compile-time type safety and memory efficiency are essential.
ArrayLists are preferred when the size of your collection varies during runtime or when you need to handle heterogeneous data types. They provide flexibility, easy initialization, and convenient element removal.
To declare and initialize an array in Java, you specify the data type, followed by square brackets and the array name, like this: int[] myArray = new int[5];
. This creates an integer array with a size of 5.
You can add elements to an ArrayList using the add()
method. For example: myArrayList.add(10);
adds the integer value 10 to the ArrayList.
No, the size of an array is fixed after initialization. If you need to change the size, you must create a new, larger array and copy the elements from the old one.
ArrayLists handle resizing automatically. When the internal capacity is reached, they allocate a new, larger array and copy elements from the old one. This resizing happens behind the scenes.
Arrays are more memory-efficient for fixed-size collections because they allocate memory precisely for the declared size. ArrayLists can consume more memory due to their dynamic resizing and reserved space for future additions.
No, ArrayLists are specific to Java and some languages that have implemented Java’s Collections Framework. Other languages may have similar dynamic array-like data structures with different names and implementations.
Read More :
Contents