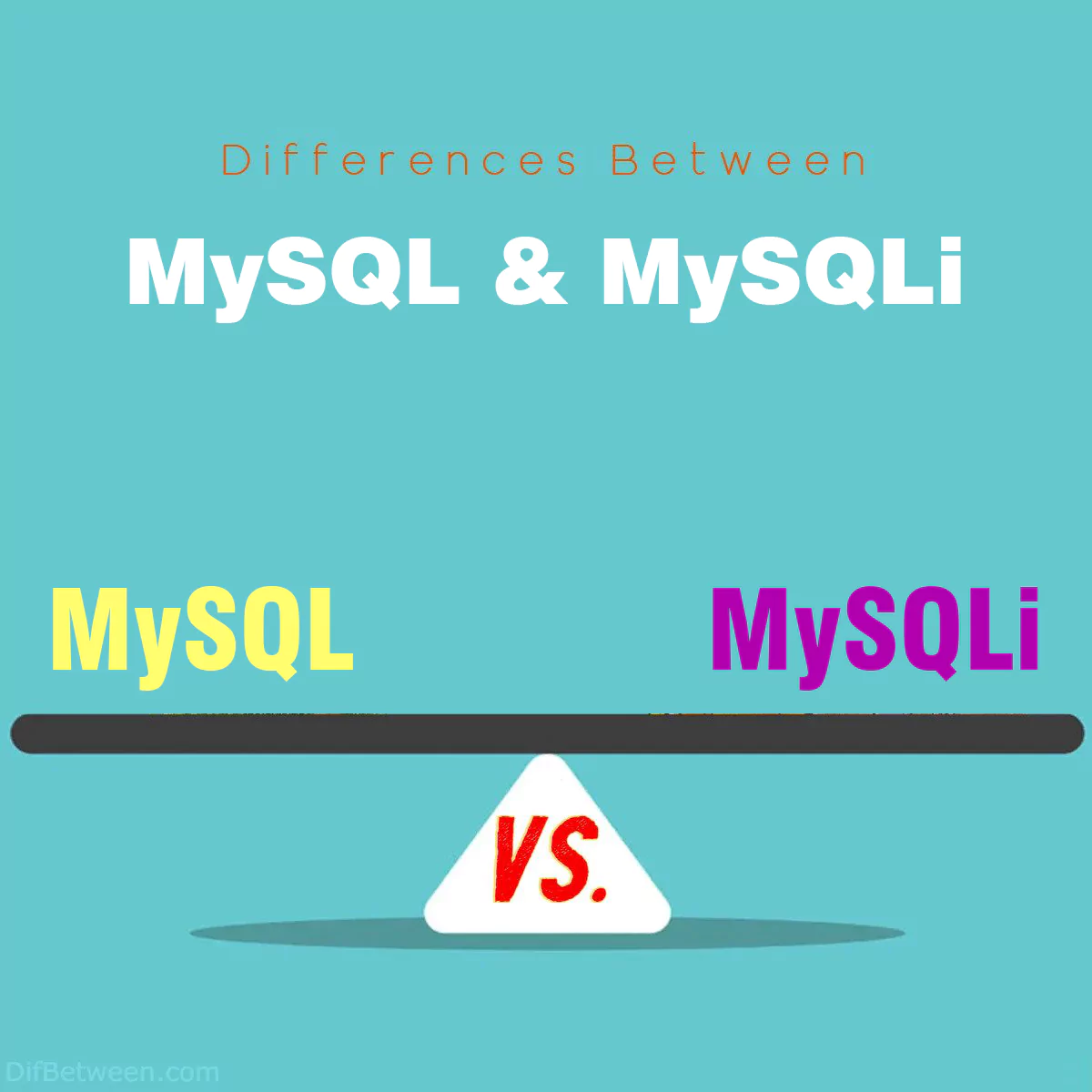
Feature | MySQL Extension (mysql ) | MySQLi Extension (mysqli ) |
---|---|---|
API Style | Primarily procedural | Both procedural and object-oriented |
Prepared Statements | Limited support (manual escaping required) | Robust support with prepared statements |
Error Handling | Limited error reporting | Enhanced error handling with detailed information |
Transaction Support | Supports transactions | Supports transactions with savepoints |
Multiple Statements in a Single Query | Not natively supported | Built-in support for executing multiple statements |
Procedural vs. Object-Oriented | Primarily procedural | Supports both procedural and object-oriented programming |
Compatibility and Future-Proofing | Deprecated and removed in PHP 7.0.0 | Actively maintained, compatible with PHP 7 and beyond |
Security | Security limitations | Enhanced security with prepared statements |
Performance | Generally performant | Performance optimizations and enhancements |
Supported Features | Limited features | Comprehensive feature set, including modern capabilities |
If you’re a developer diving into the world of databases and MySQL, you might have come across two popular terms: MySQL and MySQLi. While they may sound quite similar, they have some significant differences that can impact your database operations. In this article, we’ll be your guide to understanding the key differences between MySQL and MySQLi extensions.
Differences Between MySQL and MySQLi Extension
The main differences between MySQL and MySQLi Extension lie in their API style, security, and feature set. MySQL primarily offers a procedural API, making it suitable for quick and simple scripts but lacking advanced security features. In contrast, MySQLi provides both procedural and object-oriented programming options, making it more versatile and secure. MySQLi stands out with its robust support for prepared statements, making it a safer choice for applications that handle sensitive data. Furthermore, MySQLi offers better error handling, transaction support with savepoints, and compatibility with modern PHP versions, making it the recommended choice for new projects aiming for security, performance, and long-term viability.
1. API Style
One of the most noticeable differences between MySQL and MySQLi is the style of their APIs.
MySQL Extension
The MySQL extension (mysql
) primarily offers a procedural API. This means that you use functions like mysql_connect()
and mysql_query()
to interact with the database. Here’s an example of how you might connect to a MySQL database using the procedural style:
$connection = mysql_connect('localhost', 'username', 'password');
MySQLi Extension
On the other hand, MySQLi provides both a procedural and an object-oriented API. This gives developers more flexibility in choosing the style that suits their coding preferences. Here’s an example of connecting to a MySQL database using the object-oriented style in MySQLi:
$mysqli = new mysqli('localhost', 'username', 'password', 'database_name');
In this example, we create a new MySQLi object that represents the database connection. This object-oriented approach can make your code more organized and easier to maintain, especially for complex applications.
2. Prepared Statements
Prepared statements are a crucial feature for building secure and efficient database queries. They allow you to separate SQL code from user input, preventing SQL injection attacks and improving query execution.
MySQL Extension
The MySQL extension (mysql
) lacks native support for prepared statements. This means that you would need to manually escape and sanitize user input, which can be error-prone and less secure. Writing prepared statements in the MySQL extension can be quite challenging.
MySQLi Extension
MySQLi, on the other hand, offers robust support for prepared statements. You can easily create and execute prepared statements using either the procedural or object-oriented API. Here’s a simple example of a prepared statement in MySQLi:
$mysqli = new mysqli('localhost', 'username', 'password', 'database_name'); // Create a prepared statement $stmt = $mysqli->prepare("SELECT * FROM users WHERE username = ?"); // Bind parameters and execute $username = 'john_doe'; $stmt->bind_param('s', $username); $stmt->execute();
As you can see, MySQLi simplifies the process of working with prepared statements, making your code more secure and readable.
3. Error Handling
Effective error handling is essential in any database application. It helps you identify and resolve issues quickly.
MySQL Extension
The MySQL extension (mysql
) provides limited error handling capabilities. You can check for errors using functions like mysql_error()
, but it doesn’t offer robust support for detailed error reporting. This can make it challenging to pinpoint the exact cause of an issue when something goes wrong.
MySQLi Extension
MySQLi offers enhanced error handling features. You can access detailed error messages, error codes, and diagnostic information, making it easier to troubleshoot problems in your database queries. Here’s an example of how you can retrieve error information in MySQLi:
$mysqli = new mysqli('localhost', 'username', 'password', 'database_name'); // Check for connection errors if ($mysqli->connect_error) { die('Connection failed: ' . $mysqli->connect_error); } // Perform a query $result = $mysqli->query("SELECT * FROM non_existent_table"); // Check for query errors if (!$result) { die('Query error: ' . $mysqli->error); }
This improved error handling can save you a lot of time when debugging your PHP and MySQL applications.
4. Transaction Support
Transactions are essential for maintaining data integrity in a database. They allow you to group multiple database operations into a single unit that either succeeds entirely or fails without making partial changes to the data.
MySQL Extension
The MySQL extension (mysql
) supports transactions, but it lacks some advanced features that are available in MySQLi.
MySQLi Extension
MySQLi provides robust support for transactions and offers features like savepoints, which allow you to set markers within a transaction. This can be incredibly useful when you need to perform complex and nested database operations while maintaining data consistency.
Here’s an example of using transactions with MySQLi:
$mysqli = new mysqli('localhost', 'username', 'password', 'database_name'); // Start a transaction $mysqli->begin_transaction(); // Perform multiple database operations // Commit the transaction if everything is successful $mysqli->commit(); // Rollback the transaction if an error occurs $mysqli->rollback();
With MySQLi, you have greater control over your database transactions, ensuring data integrity in your applications.
5. Multiple Statements in a Single Query
In some cases, you may need to execute multiple SQL statements in a single query. The ability to execute multiple statements in one go can be beneficial for optimizing database operations.
MySQL Extension
The MySQL extension (mysql
) does not natively support executing multiple statements in a single query. If you attempt to execute multiple statements, it may result in an error or only execute the first statement.
MySQLi Extension
MySQLi provides built-in support for executing multiple statements in a single query. This can be done using the multi_query()
method. Here’s an example:
$mysqli = new mysqli('localhost', 'username', 'password', 'database_name'); $query = " INSERT INTO users (name, email) VALUES ('Alice', '[email protected]'); INSERT INTO users (name, email) VALUES ('Bob', '[email protected]'); "; if ($mysqli->multi_query($query)) { do { // Process each result here } while ($mysqli->next_result()); }
This feature can be particularly useful when you need to execute a batch of SQL statements efficiently.
6. Procedural vs. Object-Oriented Programming
Another significant difference between MySQL and MySQLi is the level of support for object-oriented programming (OOP).
MySQL Extension
The MySQL extension (mysql
) primarily relies on procedural programming. While you can encapsulate some functionality in functions, the overall style is procedural.
MySQLi Extension
MySQLi is designed to support both procedural and object-oriented programming. This means you can choose the programming style that aligns with your project’s architecture and your personal preferences. Developers who prefer OOP will find MySQLi’s object-oriented features more intuitive and organized.
7. Compatibility and Future-Proofing
As technology evolves, it’s essential to choose tools and libraries that are up-to-date and well-maintained to ensure the longevity of your projects.
MySQL Extension
The MySQL extension (mysql
) is considered outdated and deprecated. It was removed entirely in PHP 7.0.0, and it’s no longer actively maintained or updated. This means that if you’re using a recent version of PHP, you won’t have access to the MySQL extension.
MySQLi Extension
MySQLi, being the improved and modernized version, is actively maintained and updated. It is fully compatible with PHP 7 and beyond, ensuring that your applications will continue to work seamlessly with the latest PHP versions. Choosing MySQLi can future-proof your projects and ensure ongoing support.
8. Security
Security is a paramount concern when working with databases, as vulnerabilities can lead to data breaches and other security incidents.
MySQL Extension
The MySQL extension (mysql
) has some security limitations, especially in handling prepared statements. Developers using this extension need to be particularly cautious about SQL injection attacks and manual data sanitization.
MySQLi Extension
MySQLi offers better security features, especially with its support for prepared statements. Prepared statements, when used correctly, help prevent SQL injection attacks by automatically escaping user input. This can significantly enhance the security of your PHP and MySQL applications.
9. Performance
Database performance is critical for the responsiveness and scalability of your applications. The choice between MySQL and MySQLi can impact performance to some extent.
MySQL Extension
The MySQL extension (mysql
) has been used in countless PHP applications over the years and is generally considered performant for basic database operations. However, it may not provide the same level of optimization and performance enhancements as MySQLi.
MySQLi Extension
MySQLi includes performance improvements and optimizations over the older MySQL extension. While the performance gains may not be dramatic for simple queries, you may notice a significant difference in performance for complex queries or applications that require frequent database interactions.
10. Supported Features
Finally, let’s take a look at some additional features and capabilities that MySQLi offers over the MySQL extension.
MySQL Extension
- Limited support for transactions.
- Limited support for prepared statements.
- No support for executing multiple statements in a single query.
- Deprecated and not recommended for use in modern PHP.
MySQLi Extension
- Robust support for transactions, including savepoints.
- Full support for prepared statements, making your code more secure.
- Ability to execute multiple statements in a single query using
multi_query()
. - Actively maintained and compatible with modern PHP versions.
MySQL or MySQLi Extension: Which One is Right Choose for You?
When it comes to working with MySQL databases in PHP, choosing the right extension can significantly impact your development experience, code quality, and the overall performance of your applications. In this guide, we’ll help you decide whether MySQL or MySQLi (MySQL Improved) is the better choice for your specific needs.
MySQL Extension
When to Choose MySQL Extension:
- Legacy Projects: If you’re working on a legacy project that was developed using the MySQL extension (
mysql
) and it’s not feasible to rewrite significant portions of the code, sticking with MySQL extension might be the pragmatic choice. However, keep in mind that the MySQL extension is deprecated and not recommended for new projects. - Quick and Simple Scripts: For small, simple scripts where you don’t require advanced features like prepared statements or complex transactions, the MySQL extension can still get the job done. It’s relatively straightforward to use for basic database operations.
- Personal Familiarity: If you are already comfortable with the MySQL extension and the project doesn’t require the features offered by MySQLi, you may opt to continue using it.
MySQLi Extension
When to Choose MySQLi Extension:
- New Projects: For any new PHP project, especially those aiming for longevity and maintainability, MySQLi is the preferred choice. It offers a more modern and feature-rich API compared to the deprecated MySQL extension.
- Security Matters: Security is paramount in web development. If your application handles sensitive data or user input, MySQLi’s support for prepared statements can help you prevent SQL injection attacks effectively. This makes it a crucial choice for applications with high-security requirements.
- Object-Oriented Programming: If you prefer an object-oriented programming (OOP) approach to code organization and maintainability, MySQLi provides a more intuitive OOP interface in addition to its procedural API. This can lead to cleaner and more organized code.
- Advanced Features: If your application requires advanced database features like transactions with savepoints, executing multiple statements in a single query, or detailed error handling, MySQLi provides built-in support for these capabilities.
- Compatibility and Future-Proofing: As the MySQL extension is deprecated and has been removed in PHP 7.0.0, MySQLi is the only viable option for projects running on modern PHP versions. Choosing MySQLi ensures that your application remains compatible with the latest PHP releases.
Making the Right Choice
The decision between MySQL and MySQLi ultimately depends on your project’s specific requirements and constraints. To summarize:
- MySQL Extension: Consider it for legacy projects, quick scripts, or if you’re already familiar with it. However, be aware that it’s deprecated and not recommended for new development.
- MySQLi Extension: Opt for it in new projects, applications with security concerns, OOP-focused development, advanced features, and to ensure future compatibility with PHP versions.
In many cases, the choice is clear: MySQLi is the way to go for modern, secure, and feature-rich PHP applications. However, for existing projects that still rely on the MySQL extension, it’s essential to plan for migration to MySQLi or another modern database access method to ensure the long-term stability and security of your applications.
Remember that the success of your PHP and MySQL projects often hinges on making informed decisions about which extension to use, so choose wisely based on your project’s needs and goals.
FAQs
MySQL is an open-source relational database management system (RDBMS) known for its reliability and performance. It allows users to store, manage, and retrieve data efficiently. MySQL is often used in web applications to handle data storage and retrieval.
MySQLi stands for “MySQL Improved” and is an enhanced version of the original MySQL extension in PHP. It provides both procedural and object-oriented programming (OOP) interfaces for interacting with MySQL databases. MySQLi is designed to overcome some limitations of the old MySQL extension, offering improved security and additional features.
The main differences include:
API Style: MySQL primarily offers a procedural API, while MySQLi provides both procedural and object-oriented programming options.
Prepared Statements: MySQLi offers robust support for prepared statements, enhancing security by preventing SQL injection attacks. The MySQL extension lacks this feature.
Error Handling: MySQLi provides enhanced error handling capabilities, allowing developers to access detailed error messages and diagnostic information. The MySQL extension offers more limited error reporting.
Transaction Support: MySQLi offers advanced transaction support, including savepoints, making it more suitable for complex database operations. The MySQL extension also supports transactions but lacks some features.
For new PHP projects, it is recommended to use MySQLi. It offers better security through prepared statements, improved error handling, support for both procedural and OOP coding styles, and compatibility with modern PHP versions. Additionally, the old MySQL extension is deprecated and has been removed in PHP 7.0.0.
Yes, it is possible to migrate from MySQL to MySQLi in an existing project. However, this process may require some code refactoring, especially if your project extensively relies on the MySQL extension. It’s essential to thoroughly test the application after migration to ensure it functions correctly.
While both extensions are performant for basic database operations, MySQLi may offer better performance for complex queries and applications that require frequent database interactions. Its performance improvements and optimizations make it a suitable choice for performance-critical projects.
Yes, MySQLi is generally considered more secure than the old MySQL extension. Its support for prepared statements simplifies SQL query parameterization, which helps prevent SQL injection attacks. The MySQL extension, in contrast, relies on manual data sanitization, making it more susceptible to security vulnerabilities if not used carefully.
Yes, it is possible to use both MySQL and MySQLi in the same PHP project, but it may add complexity to your codebase. However, it’s typically not recommended unless you have a specific need for both extensions. It’s more common to choose one extension and stick with it consistently throughout the project for consistency and maintainability.
Read More :
Contents